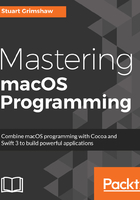
上QQ阅读APP看书,第一时间看更新
Unwrapping optionals with guard
The guard statement will only allow further execution within a scope if a test passes, and so is suitable for testing whether binding an optional to a value has succeeded:
guard let id = Int("42") else { return }
let myID: Int = id
print(myID)
The preceding code will print myID to the console, since the downcast succeeded.
Perhaps the most common usage for the guard statement is at the beginning of a function that takes an optional argument:
func printId(int: Int?)
{
guard let myInt = int else {
print(#function, "failed")
return
}
// .. do lot of code with int
print(int)
}
In the preceding code, we test for the presence of a value, and if there is nil, we print an error and then return immediately from the function. However, if there is a value in the int argument, it is unwrapped, and the rest of the function needs no extra braces, and no further unwrapping and/or testing of the myInt variable.
printId(int: Int("34a"))
What will this code print?