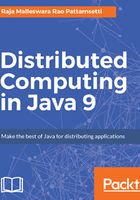
Implementing a remote interface
The next step you need to take to define the remote interface is this: implement the remote interface by a class that could interact in the RMI. When the remote interface is implemented, the constructor of this remote object needs to invoke the superclass constructor and override all the unimplemented methods.
From the infrastructure setup perspective, we should create and install a security manager, create and export one or more remote objects, and register at least one remote object with the RMI registry or Java naming convention and directory interface for reference.
The following is the CalculateEngine class implementing the Calculate remote interface:
package remote;
import java.rmi.RemoteException;
import java.rmi.server.UnicastRemoteObject;
public class CalculateEngine extends UnicastRemoteObject implements Calculate {
private static final long serialVersionUID = 1L;
public CalculateEngine() throws RemoteException {
super();
}
@Override
public long add(long parameterOne, long parameterTwo) throws
RemoteException {
return parameterOne + parameterTwo;
}
@Override
public long sub(long parameterOne, long parameterTwo) throws
RemoteException {
return parameterOne - parameterTwo;
}
@Override
public long mul(long parameterOne, long parameterTwo) throws
RemoteException {
return parameterOne * parameterTwo;
}
@Override
public long div(long parameterOne, long parameterTwo) throws
RemoteException {
return parameterOne / parameterTwo;
}
}
After you define the remote interface and implementation, write the standalone Java program through which you will start the remote server, as follows:
package remote;
import java.rmi.Naming;
import java.rmi.Remote;
public class MyServer implements Remote{
public static void main(String[] args) {
if (System.getSecurityManager() == null) {
System.setSecurityManager(new SecurityManager());
}
try {
Naming.rebind("rmi://localhost:5000/calculate",new
CalculateEngine());
System.out.println("CalculateEngine bound");
}
catch (Exception e) {
System.err.println("CalculateEngine exception:");
e.printStackTrace();
}
}
}
In the preceding program, the Naming method rebind() is used to bind the remote object with the name. The following is the list of methods Naming offers with their description:

Let's review all the steps we have taken to define the remote application:
- We declared the remote interfaces that are being implemented
- We defined a constructor for the remote object
- We provided implementations for each remote method
- We passed the objects in RMI
- We implemented the server's main method
- We created and installed a security manager
- We made the remote object available to the clients
The next step is to generate a client program that would interact with the remote object through the RMI.