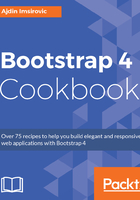
How to do it...
- Install bower using npm:
npm install –g bower
- Verify the bower installation:
which bower && bower -v
- Install Bootstrap 4 alpha 6:
bower install bootstrap#v4.0.0-alpha.6
- Install Harp globally:
npm install -g harp
- Make two new folders in the root, and add these files to them as per the following commands:
mkdir app grunt &&
touch app/index.html grunt/package.json grunt/Gruntfile.js main.scss
- Add the following code to main.scss:
$white: #ffffff;
@import "./bower_components/bootstrap/scss/bootstrap.scss";
- CD into the grunt folder:
cd grunt
- Open the package.json file and add the following code to it:
{
"name": "customgrunt",
"version": "",
"devDependencies": {
"grunt": "^1.0.1"
}
}
Then, save the file.
- In workspace/grunt, install grunt-contrib-sass:
npm install grunt-contrib-sass --save-dev
- Also, install grunt CLI (Command Line Interface):
npm install grunt-cli -g
- Add the following code to your Gruntfile.js:
'use strict';
// Load Grunt
module.exports = function (grunt) {
grunt.initConfig({
pkg: grunt.file.readJSON('package.json'),
// Tasks
sass: { // Begin Sass Plugin
dist: {
options: {
sourcemap: 'inline'
},
files: [{
expand: true,
cwd: '../',
src: ['main.scss'],
dest: '../app/css',
ext: '.css'
}]
}
},
});
// Load Grunt plugins
grunt.loadNpmTasks('grunt-contrib-sass');
// Register Grunt tasks
grunt.registerTask('default', ['sass']);
};
Then, save all files.
- The installation of grunt-cli does not install the grunt task runner. The CLI will only run Grunt if it is installed as a project dependency. We do it by running this command:
npm install grunt --save-dev
The --save-dev flag lists grunt devDependency to your package.json.
- In workspace/grunt, run the grunt command with verbose logging:
grunt -v
- Install the grunt-contrib-copy plugin:
npm install grunt-contrib-copy --save-dev
- Verify that the package.json file was updated with the new grunt plugin devDependencies; save all files.
- Update your Gruntfile.js, to include the copy task:
'use strict';
// Load Grunt
module.exports = function(grunt) {
grunt.initConfig({
pkg: grunt.file.readJSON('package.json'),
// Tasks
sass: { // Begin Sass Plugin
dist: {
options: {
sourcemap: 'inline'
},
files: [{
expand: true,
cwd: '../',
src: ['main.scss'],
dest: '../app/css',
ext: '.css'
}]
}
},
copy: {
main: {
files: [{
cwd: '../bower_components/bootstrap/dist/js/',
expand: true,
src: ['**'],
dest: '../app/js/'
}]
}
},
});
// Load Grunt plugins
grunt.loadNpmTasks('grunt-contrib-sass');
grunt.loadNpmTasks('grunt-contrib-copy');
// Register Grunt tasks
grunt.registerTask('default', ['sass', 'copy']);
};
Save the file.
- Run grunt without verbose logging:
grunt
This time, Bootstrap's JS files, bootstrap.js and bootstrap.min.js, will be copied into the app/js/ folder, as described in the new 'copy' task in our Gruntfile.js.
- Now, make sure that Harp is installed by running the version check:
harp version
- Go into the app folder and add the following code to the index.html file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-
scale=1, shrink-to-fit=no">
<meta name="description" content="">
<meta name="author" content="">
<link rel="icon" href="../../favicon.ico">
<title>Bootstrap 4 Grid Recipe</title>
<!-- Bootstrap core CSS -->
<link href="./css/main.css" rel="stylesheet" type="text/css" >
<!-- Custom styles for this template -->
<link href="starter-template.css" rel="stylesheet">
</head>
<body>
<nav class="navbar navbar-toggleable-md navbar-light bg-faded">
<button class="navbar-toggler navbar-toggler-right"
type="button" data-toggle="collapse" data-
target="#navbarsExampleDefault" aria-
controls="navbarsExampleDefault" aria-expanded="false" aria-
label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<a class="navbar-brand" href="#">Bootstrap 4 Grid Recipe</a>
<div class="collapse navbar-collapse"
id="navbarsExampleDefault">
<ul class="navbar-nav mr-auto">
<li class="nav-item active">
<a class="nav-link" href="#">Home <span class="sr-only">
(current)</span></a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Link</a>
</li>
<li class="nav-item">
<a class="nav-link disabled" href="#">Disabled</a>
</li>
<li class="nav-item dropdown">
<a class="nav-link dropdown-toggle" href="http://example.com"
id="dropdown01" data-toggle="dropdown" aria-haspopup="true"
aria-expanded="false">Dropdown</a>
<div class="dropdown-menu" aria-labelledby="dropdown01">
<a class="dropdown-item" href="#">Action</a>
<a class="dropdown-item" href="#">Another action</a>
<a class="dropdown-item" href="#">Something else here</a>
</div>
</li>
</ul>
<form class="form-inline my-2 my-lg-0">
<input class="form-control mr-sm-2" type="text"
placeholder="Search">
<button class="btn btn-outline-success my-2 my-sm-0"
type="submit">Search</button>
</form>
</div>
</nav>
<div class="container">
<div class="starter-template">
<h1>Bootstrap 4 Grid Recipe</h1>
<p class="lead">Use this document as a way to quickly start any
new project.<br> All you get is this text and a mostly barebones
HTML document.</p>
</div>
</div><!-- /.container -->
<!-- Bootstrap core JavaScript
================================================== -->
<!-- Placed at the end of the document so the pages load faster
-->
<script src="https://code.jquery.com/jquery-3.1.1.slim.min.js"
integrity="sha384-A7FZj7v+d/sdmMqp/nOQwliLvUsJfDHW+k9Omg/a/
EheAdgtzNs3hpfag6Ed950n"
crossorigin="anonymous"></script>
<script>window.jQuery || document.write('<script
src="../../assets/js/vendor/jquery.min.js"><\/script>')</script>
<script
src="https://cdnjs.cloudflare.com/ajax/libs/tether/1.4.0/js/
tether.min.js"integrity="sha384-DztdAPBWPRXSA/3eYEEUWrWCy7G5
KFbe8fFjk5JAIxUYHKkDx6Qin1DkWx51bBrb"
crossorigin="anonymous"></script>
<script src="js/bootstrap.min.js"></script>
</body>
</html>
Then, save the file.
- Navigate to the workspace/app folder, and run the following code:
harp compile
- Open the compiled www folder, and the index.html file inside. Click on the round, green Run button at the top of the Cloud9 workspace. A new tab will open next to the existing bash tab(s); there will be the Starting Apache httpd, serving text inside it. The text is followed by a clickable link, in the following format: https://<project-name>-<c9username>.c9users.io/app/www/index.html.
- Click on the provided link and a new tab will open in your browser, with the following web page:

- Go to main.scss, and change the value of the $white variable:
$white: #bada55;
Save all files.
- Now, navigate to the workspace/grunt folder, and run the grunt command:
grunt
This will recompile the .scss file and copy the .js file, from the bower_components/js/dist folder, into the app folder.
- Go to the app folder, and run harp compile again. Open the generated www/index.html and run it with the green Run button; click on the link and verify that the colors have changed to green, based on the changes made to the main.scss file.
- Now is the time to override more Bootstrap variables in the main.scss file. Above the @include, replace the override of the $white variable with the following code:
$enable-rounded: false;
$white: #ccc;
$border-width: 5px;
$font-size-base: 3rem;
Save the file. Go back to the grunt folder, and run the grunt command. Go back to the app folder, and run the harp compile command.
- Now that our setup is working, it is time to organize the Cloud9 workspace in such a way that it makes it easier for us to run the code. This will involve several simple actions. The following screenshot will give us an overview of the actions to do:

The first thing to do (marked by number 1) is click on the little icon at the top-left corner, which is the pane submenu. Next, click on the split four ways button at the bottom of the menu (marked by number 2). The third action involves dragging and dropping several open tabs, namely, index.html to the top-left corner, _custom.scss to the top-right corner, the Bash console tab that will be used to run the harp server to the lower-left corner, and finally, the bash tab that will run the grunt watch command to the lower-right corner.
- CD into the grunt folder, and install the grunt-contrib-watch grunt plugin:
npm install grunt-contrib-watch --save-dev
- Update the Gruntfile.js file with the following code:
'use strict';
// Load Grunt
module.exports = function(grunt) {
grunt.initConfig({
pkg: grunt.file.readJSON('package.json'),
// Tasks
sass: { // Begin Sass Plugin
dist: {
options: {
sourcemap: 'inline'
},
files: [{
expand: true,
cwd: '../',
src: ['main.scss'],
dest: '../app/css',
ext: '.css'
}]
}
},
copy: {
main: {
files: [
{
cwd: '../bower_components/bootstrap/dist/js/',
expand: true,
src: ['**'],
dest: '../app/js/'
}]
}
},
watch: {
files:
['../main.scss','../bower_components/bootstrap/dist/js/*.js'],
tasks: ['sass', 'copy']
},
});
// Load Grunt plugins
grunt.loadNpmTasks('grunt-contrib-sass');
grunt.loadNpmTasks('grunt-contrib-copy');
grunt.loadNpmTasks('grunt-contrib-watch');
// Register Grunt tasks
grunt.registerTask('default', ['watch']);
};
- Everything is now set up to track all the changes. In the bash tab dedicated for running grunt, type the grunt -v command. Due to the way it is set up, it will watch for changes, and report its operations with verbose logging. In the lower-left bash tab, we will start running the harp server, with the following command:
harp server ./ --port 8080
- Finally, to view your site, open a new browser tab and type the https://<project-name>-<username>.c9users.io address. This should serve the updated website. To change the look of the website, change the variable values in _custom.scss and refresh the page. With grunt watching in the lower-right console tab, and harp serving in the lower-left console tab, you only need to save changes you make to _custom.scss, and refresh the browser tab that points to your app to view the updates as they are saved.