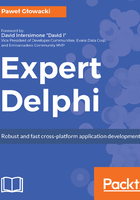
Console application
The simplest possible type of program that can be written in Delphi is a console application. It does not contain any graphical user interface. Console programs can be executed from the command prompt and can optionally take command-line parameters. A console application may output some text back to the command line, perform some calculations, process files, or communicate with remote services running somewhere on the internet.
Let's create a simple Delphi console app. It will be an interactive program that will take our name from the command line and will display a greeting. In the File menu, select New and Other. In the New Items window, make sure that the Delphi Projects node is selected, and double-click on the Console Application icon:

The IDE has generated a console application for us that currently does nothing. The first thing we always do after creating a new project is to save it. Click on Save All in the File menu, click on the Save All icon in the toolbar, or just press Ctrl + Shift + S on the keyboard:

Always save a new project into an empty folder. Enter Greeter as the name of the new project and click on OK. Notice that the IDE has changed the identifier in the first line of the code and, also, the name of the file where the program is stored. Every Delphi executable program source code needs to start from the Object Pascal keyword, program, followed by the name of the program. The name of the program should match the name of the file it is stored in. The identifier in code should match the physical file name. Otherwise, the compiler will fail to process the file.
If you now browse the folder where the project is saved, you will find two files named Greeter but with different extensions. One is the Greeter.dpr file that contains the source code of the program that we see in the Code Editor. The second one is Greeter.dproj and contains the MSBuild XML code that the IDE uses for storing different settings belonging to the project that are used for compilation. By convention, the main file of the Delphi app, the one that starts with the program keyword, does not have the.pas extension but .dpr, which stands for Delphi project.
Let's replace the content of our program with the following code:
Save and run the application. It should display an Enter your name: message and then display the welcome message. On my machine it looks as follows:
Procedures for reading and writing to console come from the built-in System unit, so in our simple demo program, there is no need for a uses clause at all. The last empty Readln statement is there in the program, so we could actually see the output from the previous Writeln statement before the program closes. Write is used to output text to the console and Read is used to read the input from the console into a string variable. The Writeln and Readln variations of these methods additionally advance to the next line in the console.
Before the begin keyword, there is a variable declaration. Object Pascal is a strongly typed language. You need to declare a variable and its type before you can use it. In our example, s is declared as string, so it can hold an arbitrary string of Unicode characters. Traditionally, in the Pascal language, the individual characters of a string can be accessed with a 1-based index. Delphi mobile compilers follow conventions used in other languages and use a 0-based indexing. There is a special compiler directive, ZEROBASEDSTRINGS, that can change this behavior.
The Code Editor helps us in a visual way to work with our code. In the screenshot from the Code Editor, on the left-hand side, in the gutter, there is a green line. It is completely green because I just saved the code. If I start typing, the color of the gutter next to the modified row will change to yellow to indicate that there are some unsaved changes. Every time you save a file, its local copy is stored in a hidden __history folder. At the bottom of the Code Editor, there is the History tab. In the History view, you can browse through changes, compare file versions, and restore a file to a previous revision.
Blue dots in the gutter indicate all executable lines of source code. This is where you can place a breakpoint if you want to debug this program. The instructions that make up the Object Pascal program start with the begin keyword and end with the end keyword, followed by .. Everything that goes between them contains statements. Each statement is separated with a semicolon (;) character. It will still be a valid program if we remove the semicolon after the last Readln instruction because the semicolon's role is to be a separator and not a terminator. The indentation of the source code and text formatting does not have any meaning for the compiler, but it is a good practice to use indentation and empty spaces for better readability. That is why the begin and end keywords start from the beginning of the line and all the other statements are indented. To increase the indentation of a block of code in the Code Editor, select the lines, keeping the Shift key pressed, and use the Ctrl + Shift + I key combination. To decrease the indentation, use Ctrl + Shift + U.
You can put arbitrary text in your source code in the form of comments, which are ignored by the compiler. Comments are multiline or single-line. Multiline comments are enclosed within curly braces ({ }) or a bracket and star character combination ((* *)). The two types of multiline comments can be nested within each other.
Some multiline comments start with a dollar sign. They are displayed in a different color in the Code Editor because they are compiler directives. In the default source code generated for a console application, there are two optional compiler directives. The first one tells the compiler that this program is a console app and the second one, with the R switch, instructs the compiler to embed in the resulting executable any binary resources that may be found in the resource file with the same name as our project, but with the .res extension.
Single-line comments start with two slashes (//). A compiler ignores all the characters that follow until it encounters the end of line control characters. If you want to quickly comment out or in a block of code, use the Ctrl + / key combination. If a comment starts with three slashes, it is treated as a special documentation comment, which can be used for displaying documentation hints in the Code Editor and documentation generation. It is a good practice to properly document your code with comments, so it is easier to read and maintain it.