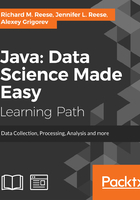
Validating dates
Many times our data validation is more complex than simply determining whether a piece of data is the correct type. When we want to verify that the data is a date for example, it is insufficient to simply verify that it is made up of integers. We may need to include hyphens and slashes, or ensure that the year is in two-digit or four-digit format.
To do this, we have created another simple method called validateDate. The method takes two String parameters, one to hold the date to validate and the other to hold the acceptable date format. We create an instance of the SimpleDateFormat class using the format specified in the parameter. Then we call the parse method to convert our String date to a Date object. Just as in our previous integer example, if the data cannot be parsed as a date, an exception is thrown and the method returns. If, however, the String can be parsed to a date, we simply compare the format of the test date with our acceptable format to determine whether the date is valid:
public static String validateDate(String theDate, String dateFormat){
try {
SimpleDateFormat format = new SimpleDateFormat(dateFormat);
Date test = format.parse(theDate);
if(format.format(test).equals(theDate)){
return theDate.toString() + " is a valid date";
}else{
return theDate.toString() + " is not a valid date";
}
} catch (ParseException e) {
return theDate.toString() + " is not a valid date";
}
}
We make the following method calls to test our method:
String dateFormat = "MM/dd/yyyy";
out.println(validateDate("12/12/1982",dateFormat));
out.println(validateDate("12/12/82",dateFormat));
out.println(validateDate("Ishmael",dateFormat));
The output follows:
12/12/1982 is a valid date
12/12/82 is not a valid date
Ishmael is not a valid date
This example highlights why it is important to consider the restrictions you place on data. Our second method call did contain a legitimate date, but it was not in the format we specified. This is good if we are looking for very specifically formatted data. But we also run the risk of leaving out useful data if we are too restrictive in our validation.