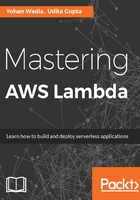
Using the CLI
As you may be well aware of, AWS provides a rich and easy to use CLI as well for managing your cloud resources. In this section, we will be using the AWS CLI to create, package, and invoke a simple Lambda function:
- To begin with, make sure your AWS CLI is set up and ready for use. You can install the CLI on most major Linux OSes, as well as macOS, and Windows. You can go through the following guide for the installation, as well as the configuration steps: http://docs.aws.amazon.com/cli/latest/userguide/installing.html.
- Next, create a new folder (in this case, I created a folder named lambda) and copy the following contents into a new file:
console.log('Loading function'); exports.handler = function(event, context) { var date = new Date().toDateString(); context.succeed("Hello " + event.username +
"! Today's date is " + date); };
The following screenshot shows the output of the preceding file:

- Name the file index.js and save it. This particular code is fairly straightforward. It logs the current date and prints a user-friendly message with the user's name passed as the event.
- Now, in order for the Lambda function to execute, we need to create a minimalistic IAM role that Lambda can assume for executing the function on our behalf. Create a file named policy.json and paste the following content into it:
{ "Version": "2012-10-17", "Statement": [ { "Effect": "Allow", "Principal": { "Service": "lambda.amazonaws.com" }, "Action": "sts:AssumeRole" } ] }
- To create an IAM Role, we need to make use of the create-role command, as shown:
# aws iam create-role --role-name basic-lambda-role --assume-role-policy-document file://policy.json
From the output of the preceding command, copy the ARN that and keep it handy. We will be requiring the same in the coming steps.

- With the role created, we can now go ahead and create our function. First, zip the index.js file using the following zip command. This is going to be our deployment package that will be uploaded to an S3 bucket and executed as a function by Lambda itself:
# zip -r mySecondFunction.zip index.js
- Next, we use the create-function command to create our Lambda function. Type in the following command:
# aws lambda create-function --region us-west-2 --function-name mySecondFunction --zip-file fileb://mySecondFunction.zip --role arn:aws:iam::00123456789:role/basic-lambda-role --handler index.handler --runtime nodejs4.3 --memory-size 128
Let us explore a few of the options that we just passed with the create-function command:
-
- --function-name: The name of the function that you will be uploading. You can optionally even provide a description for you function by passing the --description option.
- --zip-file: This is the path of the deployment package that we are uploading.
- --role: The ARN of the IAM role that Lambda will assume when it has to execute the function.
- --handler: The function name that Lambda will call to begin the execution of your code.
- --runtime: You can provide the runtime environment for the code that you will be executing. There are a few pre-defined values here that you can use, namely: nodejs, nodejs4.3, nodejs4.3-edge, java8, python2.7, and dotnetcore1.0.
- --memory-size: The amount of RAM you wish to allocate to your Lambda function. You can optionally set the timeout value as well by using the --timeout option.
The full list of options can be found here https://docs.aws.amazon.com/cli/latest/reference/lambda/create-function.html.
Once you have created the function, you should get a response similar to the one shown in the following screenshot. This means we are now ready to invoke our function:

- To invoke the function from the command line, we need to call the invoke command, as shown:
# aws lambda invoke --invocation-type RequestResponse --function-name mySecondFunction --region us-west-2 --log-type Tail --payload '{"username":"YoYo"}' output.txt
Let us explore a few of the options from the invoke command:
-
- --invocation-type: The type of invocation your Lambda will undertake. By default, the RequestResponse invocation is invoked. You can also choose between event meant for asynchronous executions or the dryrun invocation if you want to verify your function without running it.
- --log-type: Used to display the log of your function's execution. Do note, however, that the Tail parameter only works when the --invocation-type is set to RequestResponse.
- --payload: The data you wish to send to your Lambda function in JSON format.
- output: The file where you want to log the output of your function's execution.
The full specification can be found here https://docs.aws.amazon.com/cli/latest/reference/lambda/invoke.html.
- Once you have successfully invoked the function, check the output of the execution in the output.txt file:

There you have it! You have now successfully created and launched a Lambda function using the AWS CLI. There are a few other important commands that you should also keep in mind when working with the CLI, here's a quick look at some of them:
-
- list-functions: A simple command to list your published Lambda functions. You can invoke it by using the following command:
# aws lambda list-functions
You can optionally use other parameters, such as --max-items, to return a mentioned number of functions at a time:

-
- get-function and get-function-configuration: As the name suggests, both commands return the configuration information of the Lambda function that was created using the create-function command. The difference being that the get-function command also provides a unique URL to download your code's deployment package (ZIP file). The URL is pre-signed and is valid for up to 10 minutes from the time you issue the get-function command:
# aws lambda get-function <FUNCTION_NAME> (or) # aws lambda get-function-configuration
<FUNCTION_NAME>
The following screenshot shows the output for the preceding command:

To get a complete list of the CLI supported commands, click here http://docs.aws.amazon.com/cli/latest/reference/lambda/.