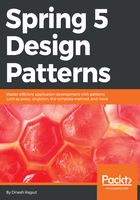
Sample implementation of the Composite design pattern
In the following example, I am implementing an Account interface, which can be either a SavingAccount and CurrentAccount or a composition of several accounts. I have a CompositeBankAccount class, which acts as a composite pattern actor class. Let's look at the following code for this example.
Create an Account interface that will be treated as a component:
public interface Account { void accountType(); }
Create a SavingAccount class and CurrentAccount class as an implementation of the component and that will also be treated as a leaf:
Following is the SavingAccount.java file:
public class SavingAccount implements Account{ @Override public void accountType() { System.out.println("SAVING ACCOUNT"); } }
Following is the CurrentAccount.java file:
public class CurrentAccount implements Account { @Override public void accountType() { System.out.println("CURRENT ACCOUNT"); } }
Create a CompositeBankAccount class that will be treated as a Composite and implements the Account interface:
Following is the CompositeBankAccount.java file:
package com.packt.patterninspring.chapter3.composite.pattern; import java.util.ArrayList; import java.util.List; import com.packt.patterninspring.chapter3.model.Account; public class CompositeBankAccount implements Account { //Collection of child accounts. private List<Account> childAccounts = new ArrayList<Account>(); @Override public void accountType() { for (Account account : childAccounts) { account.accountType(); } } //Adds the account to the composition. public void add(Account account) { childAccounts.add(account); } //Removes the account from the composition. public void remove(Account account) { childAccounts.remove(account); } }
Create a CompositePatternMain class that will also be treated as a Client:
Following is the CompositePatternMain.java file:
package com.packt.patterninspring.chapter3.composite.pattern; import com.packt.patterninspring.chapter3.model.CurrentAccount; import com.packt.patterninspring.chapter3.model.SavingAccount; public class CompositePatternMain { public static void main(String[] args) { //Saving Accounts SavingAccount savingAccount1 = new SavingAccount(); SavingAccount savingAccount2 = new SavingAccount(); //Current Account CurrentAccount currentAccount1 = new CurrentAccount(); CurrentAccount currentAccount2 = new CurrentAccount(); //Composite Bank Account CompositeBankAccount compositeBankAccount1 = new
CompositeBankAccount(); CompositeBankAccount compositeBankAccount2 = new
CompositeBankAccount(); CompositeBankAccount compositeBankAccount = new
CompositeBankAccount(); //Composing the bank accounts compositeBankAccount1.add(savingAccount1); compositeBankAccount1.add(currentAccount1); compositeBankAccount2.add(currentAccount2); compositeBankAccount2.add(savingAccount2); compositeBankAccount.add(compositeBankAccount2); compositeBankAccount.add(compositeBankAccount1); compositeBankAccount.accountType(); } }
Let's run this demo class and see the following output at the console:

Now that we have discussed the composite design pattern, let's turn to the decorator design pattern.