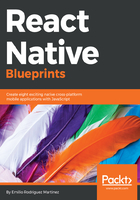
The store
MobX uses the concept of "observable" properties. We should declare an object containing our general application's state, which will hold and declare those observable properties. When we modify one of these properties, all the subscribed observers will be updated by MobX automatically. This is the basic principle behind MobX, so let's take a look at a sample code:
/*** src/store.js ***/
import {observable} from 'mobx';
class Store {
@observable feeds;
...
constructor() {
this.feeds = [];
}
addFeed(url, feed) {
this.feeds.push({
url,
entry: feed.entry,
title: feed.title,
updated: feed.updated
});
this._persistFeeds();
}
...
}
const store = new Store()
export default store
We have an attribute, feeds, marked as @observable, meaning that any component can subscribe to it and be notified every time the value is changed. This attribute is initialized as an empty array in the class constructor.
Finally, we also created the addFeed method, which will push a new feed into the feeds attribute and therefore will trigger automatic updates on all the observers. To better understand MobX observers, let's take a look at a sample component observing the feeds list:
import React from 'react';
import { Container, Content, List, ListItem, Text } from 'native-base';
import { observer } from 'mobx-react/native';
@observer
export default class FeedsList extends React.Component {
render() {
const { feeds } = this.props.screenProps.store;
return (
<Container>
<Content>
<List>
{feeds &&
feeds.map((f, i) => (
<ListItem key={i}>
<Text>{f.title}</Text>
</ListItem>
))}
</List>
</Content>
</Container>
);
}
}
The first thing we notice is the need to mark our component with the @observer decorator to ensure it is updated when any of the @observable properties change in our store.
Another thing to note is the need for the store to be received in the component as a property. In this case, since we are using react-navigation, we will pass it inside screenProps, which is the standard way in react-navigation for sharing properties between <Navigator> and its child screens.
MobX has many more features, but we will leave those for more complex apps as one of the goals for this chapter is to show how simple state management can be when we are building small apps.