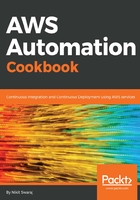
How to do it...
We can initiate the project by hitting yarn init:
[root@awsstar ~]# mkdir example-node-server
[root@awsstar ~]# cd example-node-server
[root@awsstar ~]# yarn init
yarn init v0.27.5
question name (example-node-server): example-node-server
question version (1.0.0):
question description: sample nodejs webapp
question entry point (index.js): lib/index.js
question repository url:
question author: Nikit Swaraj
question license (MIT): MIT
success Saved package.json
Done in 53.06s.
In the preceding output of execution of the yarn init command, we get some parameter where we have to fill some input from our end. After initiating the project, we will get a file called package.json.
package.json is a file where we can fill the dependency's name and build step or set some script to get executed. At this moment, we will see the parameter values, which we entered while initiating the project:
[root@awsstar example-node-server]# ls
package.json
[root@awsstar example-node-server]# cat package.json
{
"name": "example-node-server",
"version": "1.0.0",
"description": "sample nodejs webapp",
"main": "lib/index.js",
"author": "Nikit Swaraj",
"license": "MIT"
}
Let's write some basic code:
[root@awsstar example-node-server]# mkdir lib && cd lib
[root@awsstar example-node-server]# vi index.js
import http from 'http';
http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello AWSSTAR\n');
}).listen(1337, '127.0.0.1');
console.log('Server running at http://127.0.0.1:1337/');
:wq (save and quite)
Write one small test case, which will check 200 status OK:
[root@awsstar example-node-server]# mkdir test && cd test
[root@awsstar example-node-server]# vi index.js
import http from 'http';
import assert from 'assert';
import '../lib/index.js';
describe('Example Node Server', () => {
it('should return 200', done => {
http.get('http://127.0.0.1:1337', res => {
assert.equal(200, res.statusCode);
done();
});
});
});
:wq (save and quit)
We will use some commands to build the application, which are dependencies. So, we have to tweak the package.json file by mentioning the dependencies and required scripts:
[root@awsstar example-node-server]# vi package.json
{
"name": "example-node-server",
"version": "1.0.0",
"description": "sample nodejs webapp",
"main": "lib/index.js",
"scripts": {
"start": "nodemon lib/index.js --exec babel-node --presets es2015,stage-2",
"build": "babel lib -d dist",
"serve": "node dist/index.js",
"test": "mocha --compilers js:babel-register"
},
"author": "Nikit Swaraj",
"license": "MIT",
"devDependencies": {
"babel-cli": "^6.24.1",
"babel-preset-es2015": "^6.24.1",
"babel-preset-stage-2": "^6.24.1",
"babel-register": "^6.11.6",
"mocha": "^3.0.1",
"nodemon": "^1.11.0"
}
}
Once we fill all the dependencies and necessary scripts, its time to install the dependencies, build the application, run it, and test it.