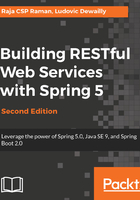
updateUser – implementation in the handler and repository
Here, we will define and implement the updateUser method in our repository. Also, we will call the updateUser method in the main class through UserHandler.
We will add an abstract method for the updateUser method in the UserRepository class:
Mono<Void> updateUser(Mono<User> userMono);
In the UserRepositorySample class, we will add the logic to update the code. Here, we will use the userid as the key and the User object as the value to store in our map:
@;Override
public Mono<Void> updateUser(Mono<User> userMono) {
return userMono.doOnNext(user -> {
users.put(user.getUserid(), user);
System.out.format("Saved %s with id %d%n", user, user.getUserid());
}).thenEmpty(Mono.empty());
}
In the preceding code, we have updated the user by adding the specified user (from the request). Once the user is added in the list, the method will return Mono<Void>; otherwise, it will return the Mono.empty object.
As we have added the updateUser method in the repository, here we will follow up on our handler:
public Mono<ServerResponse> updateUser(ServerRequest request) {
Mono<User> user = request.bodyToMono(User.class);
return ServerResponse.ok().build(this.userRepository.saveUser(user));
}
In the preceding code, we have converting the user request to Mono<User> by calling the bodyToMono method. The bodyToMono method will extract the body into a Mono object, so it can be used for the saving option.
As we did with other API paths, we add the updateUser API in Server.java:
public RouterFunction<ServerResponse> routingFunction() {
UserRepository repository = new UserRepositorySample();
UserHandler handler = new UserHandler(repository);
return nest (
path("/user"),
nest(
accept(MediaType.ALL),
route(GET("/"), handler::getAllUsers)
)
.andRoute(GET("/{id}"), handler::getUser)
.andRoute(POST("/").and(contentType(APPLICATION_JSON)), handler::createUser)
.andRoute(PUT("/").and(contentType(APPLICATION_JSON)), handler::updateUser)
);
}