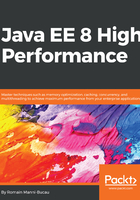
@Suspended or asynchronous operation
JAX-RS 2.1 got a brand new reactive API to integrate with Java 8 CompletionStage but the server also has a nice integration to be reactive: @Suspended. For instance, the findAll method of QuoteResource could look like the following:
@Path("quote")
@RequestScoped
public class QuoteResource {
@Inject
private QuoteService quoteService;
@Resource
private ManagedExecutorService managedExecutorService;
@GET
public void findAll(@Suspended final AsyncResponse response, <1>
@QueryParam("from") @DefaultValue("0") final int from,
@QueryParam("to") @DefaultValue("10") final int to) {
managedExecutorService.execute(() -> { <2>
try {
final long total = quoteService.countAll();
final List<JsonQuote> items = quoteService.findAll(from, to)
.map(quote -> {
final JsonQuote json = new JsonQuote();
json.setId(quote.getId());
json.setName(quote.getName());
json.setValue(quote.getValue());
json.setCustomerCount(ofNullable(quote.getCustomers())
.map(Collection::size).orElse(0));
return json;
})
.collect(toList());
final JsonQuotePage page = new JsonQuotePage();
page.setItems(items);
page.setTotal(total);
response.resume(page); <3>
} catch (final RuntimeException re) {
response.resume(re); <3>
}
});
}
// ...
}
In the synchronous flavor of a JAX-RS method, the returned instance is the response payload. However, when going asynchronous, the returned instance is no more used as the payload in JAX-RS 2.0; the only option is to use the AsyncResponse JAX-RS API to let the container be notified of the state of processing of the request. Since JAX-RS 2.1 (Java EE 8), you can also return a Java 8 CompletionStage instance, which gives you the same hooks, and the server can integrate with it to be notified of the success or failure of the invocation. In any case, both kinds of APIs imply the same kind of logic:
- The @Suspended annotation marks a parameter of the AsyncResponse type to be injected. This is the callback holder you use to notify JAX-RS that you have finished the execution and have made JAX-RS resume the HTTP request. If you use the CompletionStage API flavor, you don't need this parameter and can directly use your CompletionStage instance almost the same way.
- This asynchronous API makes sense when the computation of the response is asynchronous. So, we need to submit the task in a thread pool. In EE 8 the best way to do it correctly is to rely on the EE concurrency utility API and, therefore, ManagedExecutorService.
- Once the computation is finished, resume() is used to send back the response (normal payload or throwable), which will use ExceptionMappers to be translated in payload.
With this pattern, you need to take into account the fact that there is another thread pool apart from the HTTP one. It will impact at different levels, which we will deal with later, but an important point is that increasing the number of threads doesn't mean improving the performance in all cases, and for fast execution, you can even decrease your performance.