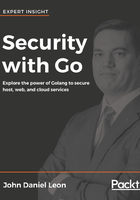
switch, case, fallthrough, and default
The switch statement allows you to branch execution based on the state of a variable. It is similar to the switch statement in C and other languages.
There is no fallthrough by default. This means once the end of a case is reached, the code exits the switch statement completely unless an explicit fallthrough command is provided. A default case can be provided if none of the cases are matched.
You can put a statement in front of the variable to be switched, such as the if statement. This creates a variable whose scope is limited to the switch statement.
This example demonstrates two switch statements. The first one uses hardcoded values and includes a default case. The second switch statement uses an alternate syntax that allows for a statement in the first line:
package main
import (
"fmt"
"math/rand"
)
func main() {
x := 42
switch x {
case 25:
fmt.Println("X is 25")
case 42:
fmt.Println("X is the magical 42")
// Fallthrough will continue to next case
fallthrough
case 100:
fmt.Println("X is 100")
case 1000:
fmt.Println("X is 1000")
default:
fmt.Println("X is something else.")
}
// Like the if statement a statement
// can be put in front of the switched variable
switch r := rand.Int(); r {
case r % 2:
fmt.Println("Random number r is even.")
default:
fmt.Println("Random number r is odd.")
}
// r is no longer available after the switch statement
}