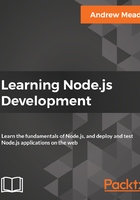
Trying and catching code block
Now, if the notes-data.json file does not exist, which it won't when the user first runs the command, the program will crash, as shown in the following code output. We can prove this by simply rerunning the last command after deleting the note-data.JSON file:

Right here, you can see we're actually getting a JavaScript error, no such file or directory; it's trying to open up the notes-data.JSON file, but without much success. To fix this, we'll use a try-catch statement from JavaScript, which hopefully you've seen in the past. To brush up this, let's go over it really quick.
To create a try-catch statement, all you do is you type try, which is a reserved keyword, and then you open and close a set of curly braces. Inside the curly braces is the code that will run. This is the code that may or may not throw an error. Next, you'll specify the catch block. Now, the catch block will take an argument, an error argument, and it also has a code block that runs:
try{
} catch (e) {
}
This code will run if and only if one of your errors in try actually occurs. So, if we load the file using readFileSync and the file exists, that's fine, catch block will never run. If it fails, catch block will run and we can do something to recover from that error. With this in place, all we'll do is move the noteString variable and the JSON.parse statements into try, as shown here:
try{
var notesString = fs.readFileSync('notes-data.json');
notes = JSON.parse(notesString);
} catch (e) {
}
That's it; nothing else needs to happen. We don't need to put any code in catch, although you do need to define the catch block. Now, let's take a look at what happens when we run the whole code.
The first thing that happens is that we create our static variables—nothing special there—then we try to load in the file. If the notesString function fails, that is fine because we already defined notes to be an empty array. If the file doesn't exist and it fails, then we probably want an empty array for notes anyways, because clearly there are no notes, and there's no file.
Next up, we'll parse that data into notes. There is a chance that this will fail if there's invalid data in the notes-data.JSON file, so the two lines can have problems. By putting them in try-catch, we're basically guaranteeing that the program isn't going to work unexpectedly, whether the file does or doesn't exist, but it contains corrupted data.
With this in place, we can now save notes and rerun that previous command. Note that I do not have the notes-data file in place. When I run the command, we don't see any errors, everything seems to run as expected:

When you now visit Atom, you can see that the notes-data file does indeed exist, and the data inside it looks great:

This is all we need to do to fetch the notes, update the notes with the new note, and finally save the notes to the screen.
Now, there is still a slight problem with addNote. Currently, addNote allows for duplicate titles; I could already have a note in the JSON file with the title of secret. I can come along and try to add a new note with the title of secret and it will not throw an error. What I'd like to do is to make the title unique, so that if there's already a note with that title, it will throw an error, letting you know that you need to create a note with a different title.