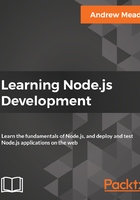
Getting the specific note information
Now, what we did in the previous subsection is step 1. We now have support for various commands. The next thing we need to figure out is how we'll get more specific information. For example, which note do you want to remove? Which note do you want to read? And what do you want the note text to be in the case of adding a note? This is all information we need to get from the Terminal.
Now, getting it is going to be pretty similar to what we did earlier, and to show you what it looks like, we'll print the entire argv object once again, using the following command:
console.log(process.argv);
Over in the Terminal, we can now run a more complex command. Let's say we want to remove a note using the node app.js remove command, and we'll do that by its title. We might use the title argument, which looks like the following code:
node app.js remove --title
In this title argument, we have -- (two) hyphens followed by the argument name, which is title, followed by the = (equals) sign. Then we can type our note title. Maybe the note title is secrets. This will pass the title argument into our application.
Now, there are a couple of different ways you could format the title argument, which are as follows:
- You could have the title secrets like the one in the preceding command
- You could have title equals secrets inside quotes, which will let us use spaces in the title:
node app.js remove --title=secrets
- You can remove the = (equals) sign altogether and simply put a space:
node app.js remove --title="secrets 2"
No matter how you choose to format your argument, these are all valid ways to pass in the title.
For the moment, I'll keep the = (equals) sign and the quotes and rerun the command:
node app.js remove --title="secrets 2"
When I run the command, you can see in the following code output that we have our two arguments:

These are the arguments that we don't need, then we have our remove command, which is the third one, and we now have a new fourth string, the title that is equal to secrets 2. And our argument was successfully passed into the application. The problem is that it's not very easy to use. In the fourth string, we have to parse out the key, which is title, and the value, which is secrets 2.
When we used the command, which was the third argument in the previous section, it was a lot easier to use inside our app. We simply pulled it out of the arguments array and we referenced it by using the command variable and checking whether it equaled add, list, read, or remove.
Things get a lot more complex as we use different styles for passing in the arguments. If we rerun the last command with a space instead of an = (equals) sign, as shown in the following code, which is perfectly valid, our arguments array now looks completely different:

In the preceding code output, you can see that we have the title as the fourth item, and we have the value, which is secrets 2, as the fifth, which means we have to add other conditions for parsing. And this turns into a pain really quickly, which is why we will not do it.
We'll use a third-party module called yargs in the next chapter to make parsing the command-line arguments effortless. Instead of having strings, as shown in this one or the one we discussed earlier, we'll get an object where the title property equals the secrets 2 string. That will make it super easy to implement the rest of the notes application.
Now, parsing certain types of command-line arguments, such as key value pairs, becomes a lot more complex, which is why, in the next chapter, we'll be using yargs to do just that.