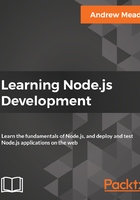
Creating projects using npm modules
Now, in the npm packages, there's nothing magical, it's regular Node code that aims to solve a specific problem. The reason you'd want to use it is so you don't have to spend all your time writing these utility functions that already exist; not only do they exist, they've been tested, they've been proven to work, and others have used them and documented them.
Now, with all that said, how do we get started? Well, to get started, we actually have to run a command from the Terminal to tell our application we want to use npm modules. This command will be run over in the Terminal. Make sure you've navigated inside your project folder and inside the notes-node directory. Now, when you installed Node, you also installed something called npm.
We will be running some npm commands and you can test that you have it installed by running npm, a space, and -v (we're running npm with the v flag). This should print the version, as shown in the following code:

It's okay if your version is slightly different, that's not important; what is important is that you have npm installed.
Now, we'll run a command called npm init in Terminal. This command will prompt us to fill out a few questions about our npm project. We can run the command and we can cycle through the questions, as shown in the following screenshot:

In the preceding screenshot, at the top is a quick description of what's happening, and down below it'll start asking you a few questions, as shown in the following screenshot:

The questions include the following:
- name: Your name can't have uppercase characters or spaces; you can use notes-node, for example. You can hit enter to use the default value, which is in parentheses.
- version: 1.0.0 works fine too; we will leave most of these at their default value.
- description: We can leave this empty at the moment.
- entry point: This will be app.js, make sure that shows up properly.
- test command: We'll explore testing later in the book, so for now, we can leave this empty.
- git repository: We'll leave that empty for now as well.
- keywords: These are used for searching for modules. We'll not be publishing this module so we can leave those empty.
- author: You might as well type your name.
- license: For the license, we'll stick with ISC at the moment; since we're not publishing it, it doesn't really matter.
After answering these questions, if we hit enter, we'll get the following on our screen and a final question:

Now, I want to dispel the myth that this command is doing anything magical. All this command is doing is creating a single file inside your project. It'll be in the root of the project and it's called package.json, and the file will look exactly like the preceding screenshot.
To the final question, as shown down below in the preceding image, you can hit enter or type yes to confirm that this is what you want to do:

Now that we have created the file, we can actually view it inside our project. As shown in the following code, we have the package.json file:
{
"name": "notes-node",
"version": "1.0.0",
"description": "",
"main": "app.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC"
}
And this is all it is, it's a simple description of your application. Now, as I mentioned, we'll not be publishing our app to npm, so a lot of this information really isn't important to us. What is important, though, is that package.json is where we define the third-party modules we want to install in our application.