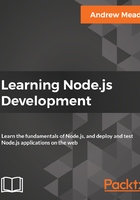
Creating and appending files in the File System module
To kick things off though, we will start with the File System module. We'll go through the process of creating a file and appending to it:

When you view a docs page for a built-in module, whether it's File System or a different module, you'll see a long list of all the different functions and properties that you have available to you. The one we'll use in this section is fs.appendFile.
If you click on it, it will take you to the specific documentation, and this is where we can figure out how to use appendFile, as shown in the following screenshot:

Now, appendFile is pretty simple. We'll pass to it two string arguments (shown in the preceding screenshot):
- One will be the file name
- The other will be the data we want to append to the file
This is all we need to provide in order to call fs.appendFile. Before we can call fs.appendFile, we need to require it. The whole point of requiring is to let us load in other modules. In this case, we'll load in the fs module from app.js.
Let's create a variable that will be a constant, using const.
Then we'll give it a name, fs and set it equal to require(), as shown in the following code:
const fs = require()
Here, require() is a function that's available to you inside any of your Node.js files. You don't have to do anything special to call it, you simply call it as shown in the preceding code. Inside the argument list, we'll just pass one string.
In our case, we'll pass in the module name, which is fs and toss in a semicolon at the end, as shown in the following code:
const fs = require('fs');
This will tell Node that you want to fetch all of the contents of the fs module and store them in the fs variable. At this point, we have access to all of the functions available on the fs module, which we explored over in the docs, including fs.appendFile.
Back in Atom, we can call the appendFile by calling fs.appendFile, passing in the two arguments that we'll use; the first one will be the filename, so we add greetings.txt, and the second one will be the text you want to append to the file. In our case, we'll append Hello world!, as shown in the following code:
fs.appendFile('greetings.txt', 'Hello world!');
Let's save the file, as shown in the preceding command, and run it from Terminal to see what happens.
If you're running Node v7 or greater, you'll get a little warning when you run the program inside Terminal. Now, on v7, it'll still work, it's just a warning, but you can get rid of it using the following code:
// Orignal line
fs.appendFile('greetings.txt', 'Hello world!');
// Option one
fs.appendFile('greetings.txt', 'Hello world!', function (err){
if (err) {
console.log('Unable to write to file');
}
});
// Option two
fs.appendFileSync('greetings.txt', 'Hello world!');
In the preceding code, we have the original line that we have inside our program.
In Option one here is to add a callback as the third argument to the append file. This callback will get executed when either an error occurs or the file successfully gets written too. Inside option one, we have an if statement; if there is an error, we simply print a message to the screen, Unable to write to file.
Now, our second option in the preceding code, Option two, is to call appendFileSync, which is a synchronous method (we'll talk more about that later); this function does not take the third argument. You can type it as shown in the preceding code and you won't get the warning.
So, pick one of these two options if you see the warning; both will work much the same.
If you are on v6, you can stick with the the original line, shown at the top of the preceding code, although you might as well use one of the two options below that line to make your code a little more future proof.
Fear not, we'll be talking about asynchronous and synchronous functions, as well as callback functions, extensively throughout the book. What I'm giving you here in the code is just a template, something you can write in your file to get that error removed. In a few chapters, you will understand exactly what these two methods are and how they work.
If we do the appending over in Terminal, node app.js, we'll see something pretty cool:

As shown in the preceding code, we get our one console.log statement, Starting app.. So we know the app started correctly. Also, if we head over into Atom, we'll actually see that there's a brand new greetings.txt file, as shown in the following code. This is the text file that was created by fs.appendFile:
console.log('Starting app.');
const fs = require('fs');
fs.appendFile('greetings.txt', 'Hello world!');
Here, fs.appendFile tries to append greetings.txt to a file; if the file doesn't exist, it simply creates it:

You can see that we have our message, Hello world! in the greetings.txt file, printing to the screen. In just a few minutes, we were able to load in a built-in Node module and call a function that lets us create a brand new file.
If we call it again by rerunning the command using the up arrow key and the enter key, and we head back into the contents of greetings.txt, you can see this time around that we have Hello world! twice, as shown here:

It appended Hello world! one time for each time we ran the program. We have an application that creates a brand new file on our filesystem, and if the file already exists, it simply adds to it.