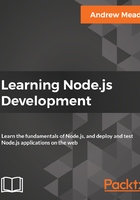
The working of blocking I/O
The blocking example can be illustrated as follows:

The first thing that happens inside our blocking example, as shown in the preceding screenshot, is that we fetch the user on line 3 in the code:
var user1 = getUserSync('123');
Now, this request requires us to go to a database, which is an I/O operation to fetch that user by ID. This takes a little bit of time. In our case, we'll say it takes three seconds.
Next, on line 4 in the code, we print the user to the screen, which is not an I/O operation and it runs right away, printing user1 to the screen, as shown in the following code:
console.log('user1', user1);
As you can see in the following screenshot, it takes almost no time at all:

Next up, we wait on the fetching of user2:
var user2 = getUserSync('321');

When user2 comes back, as you might expect, we print it to the screen, which is exactly what happens on line 7:
console.log('user2', user2);
Finally, we add up our numbers and we print it to the screen:
var sum = 1 + 2; console.log('The sum is ' + sum);
None of this is I/O, so right here we have our sum printing to the screen in barely any time.
This is how blocking works. It's called blocking because while we're fetching from the database, which is an I/O operation, our application cannot do anything else. This means our machine sits around idle waiting for the database to respond, and can't even do something simple like adding two numbers and printing them to the screen. It's just not possible in a blocking system.