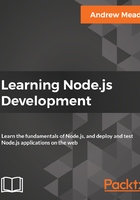
Using the getNote function
Let's work on this method. Now, the first thing I'll do is fill out, inside of app.js, a variable called note which is going to store the return value from getNote:
} else if (command === 'read') {
var note = notes.getNote(argv.title);
Now, this could be an individual note object or it could be undefined. In the next line, I can use an if statement to print the message if it exists, or if it does not exist. I'll use if note, and I am going to attach an else clause:
} else if (command === 'read') {
var note = notes.getNote(argv.title);
if (note) {
} else {
}
This else clause will be responsible for printing an error if the note is not found. Let's get started with that first since it's pretty simple, console.log, Note not found, as shown here:
if (note) {
} else {
console.log('Note not found');
}
Now that we have our else clause filled out we can fill out the if statement. For this, I'll print a little message, console.log ('Note found') will get the job done. Then we can move on to printing the actual note details, and we already have that code in place. We are going to add the hyphenated spacer, then we have our note title and our note body as shown here:
if (note) {
console.log('Note found');
console.log('--');
console.log(`Title: ${note.title}`);
console.log(`Body: ${note.body}`);
} else {
console.log('Note not found');
}
Now that we're done with the inside of app.js, we can move into the notes.js file and fill out the getNote method because currently it doesn't do anything with the title that gets passed in.
Inside notes, what you needed to do was fill out those three lines. The first one is going to be responsible for fetching the notes. We already have did that before with the fetchNotes function in the previous section:
var getNote = (title) => {
var notes = fetchNotes();
};
Now that we have our notes in place we, can call notes.filter, returning all of the notes. I'll make a variable called filteredNotes, setting it equal to notes.filter. Now, we know that the filter method takes a function, I'll define an arrow function (=>) just like this:
var filteredNotes = notes.filter(() => {
});
Inside the arrow function (=>), we'll get the individual note passed in, and we'll return true when the note title, the title of the note we found in our JSON file, equals, using triple equals, title:
var filteredNotes = notes.filter(() => {
return note.title === title;
});
};
This will return true when the note title matches and false if it doesn't. Alternatively, we can use arrow functions, and we only have one line, as shown following, where we return something; we can cut out our condition, remove the curly braces, and simply paste that condition right here:
var filteredNotes = notes.filter((note) => note.title === title);
This has the exact same functionality, only it's a lot shorter and easier to look at.
Now that we have all of the data, all we need to do is return something, and we'll return the first item in the filteredNotes array. Next, we'll grab the first item, which is the index of zero, and then we just need to return it using the return keyword:
var getNote = (title) => {
var notes = fetchNotes();
var filteredNotes = notes.filter((note) => note.title === title);
return filteredNotes[0];
};
Now, there is a chance that filteredNotes, the first item, doesn't exist, and that's fine, it's going to return undefined, in which case our else clause will run, printing Note not found. If there is a note, great, that's the note we want to print, and over in app.js we do just that.