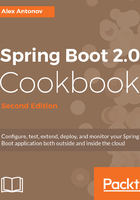
上QQ阅读APP看书,第一时间看更新
How to do it...
- Create a new package folder named entity under the src/main/java/com/example/bookpub directory from the root of our project.
- In this newly created package, create a new class named Book with the following content:
@Entity public class Book { @Id @GeneratedValue private Long id; private String isbn; private String title; private String description; @ManyToOne private Author author; @ManyToOne private Publisher publisher; @ManyToMany private List<Reviewers> reviewers; protected Book() {} public Book(String isbn, String title, Author author,
Publisher publisher) { this.isbn = isbn; this.title = title; this.author = author; this.publisher = publisher; } //Skipping getters and setters to save space, but we do need them }
- As any book should have an author and a publisher, and ideally some reviewers, we need to create these entity objects as well. Let's start by creating an Author entity class, under the same directory as our Book, as follows:
@Entity public class Author { @Id @GeneratedValue private Long id; private String firstName; private String lastName; @OneToMany(mappedBy = "author") private List<Book> books; protected Author() {} public Author(String firstName, String lastName) {...} //Skipping implementation to save space, but we do need
it all }
- Similarly, we will create the Publisher and Reviewer classes, as shown in the following code:
@Entity public class Publisher { @Id @GeneratedValue private Long id; private String name; @OneToMany(mappedBy = "publisher") private List<Book> books; protected Publisher() {} public Publisher(String name) {...} } @Entity public class Reviewer { @Id @GeneratedValue private Long id; private String firstName; private String lastName; protected Reviewer() {} public Reviewer(String firstName, String lastName)
{...}
}
- Now we will create our BookRepository interface by extending Spring's CrudRepository interface under the src/main/java/com/example/bookpub/repository package, as follows:
@Repository public interface BookRepository
extends CrudRepository<Book, Long> { public Book findBookByIsbn(String isbn); }
- Finally, let's modify our StartupRunner class in order to print the number of books in our collection, instead of some random datasource string, by autowiring a newly created BookRepository and printing the result of a .count() call to the log, as follows:
public class StartupRunner implements CommandLineRunner { @Autowired private BookRepository bookRepository; public void run(String... args) throws Exception { logger.info("Number of books: " +
bookRepository.count()); } }