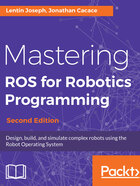
Building the nodes
We have to edit the CMakeLists.txt file in the package to compile and build the source code. Navigate to mastering_ros_demo_pkg to view the existing CMakeLists.txt file. The following code snippet in this file is responsible for building these two nodes:
include_directories( include ${catkin_INCLUDE_DIRS} ${Boost_INCLUDE_DIRS} ) #This will create executables of the nodes add_executable(demo_topic_publisher src/demo_topic_publisher.cpp) add_executable(demo_topic_subscriber src/demo_topic_subscriber.cpp) #This will generate message header file before building the target add_dependencies(demo_topic_publisher mastering_ros_demo_pkg_generate_messages_cpp) add_dependencies(demo_topic_subscriber mastering_ros_demo_pkg_generate_messages_cpp) #This will link executables to the appropriate libraries target_link_libraries(demo_topic_publisher ${catkin_LIBRARIES}) target_link_libraries(demo_topic_subscriber ${catkin_LIBRARIES})
We can add the preceding snippet to create a new a CMakeLists.txt file for compiling the two codes.
The catkin_make command is used to build the package. We can first switch to a workspace:
$ cd ~/catkin_ws
Build mastering_ros_demo_package as follows:
$ catkin_make
We can either use the preceding command to build the entire workspace, or use the the -DCATKIN_WHITELIST_PACKAGES option. With this option, it is possible to set one or more packages to compile:
$ catkin_make -DCATKIN_WHITELIST_PACKAGES="pkg1,pkg2,..."
Note that is necessary to revert this configuration to compile other packages or the entire workspace. This can be done using the following command:
$ catkin_make -DCATKIN_WHITELIST_PACKAGES=""
If the building is done, we can execute the nodes. First, start roscore:
$ roscore
Now run both commands in two shells. In the running publisher:
$ rosrun mastering_ros_demo_package demo_topic_publisher
In the running subscriber:
$ rosrun mastering_ros_demo_package demo_topic_subscriber
We can see the output as shown here:

The following diagram shows how the nodes communicate with each other. We can see that the demo_topic_publisher node publishes the /numbers topic and then subscribes to the demo_topic_subscriber node:

We can use the rosnode and rostopic tools to debug and understand the working of two nodes:
- $ rosnode list: This will list the active nodes.
- $ rosnode info demo_topic_publisher: This will get the info of the publisher node.
- $ rostopic echo /numbers: This will display the value sending through the /numbers topic.
- $ rostopic type /numbers: This will print the message type of the /numbers topic.