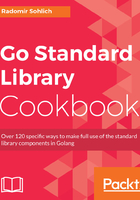
上QQ阅读APP看书,第一时间看更新
How to do it...
- Open the console and create the folder chapter02/recipe08.
- Navigate to the directory.
- Create the file win1250.txt with content Gdańsk. The file must be encoded in the windows-1250 charset. If you are not sure how to do that, just jump to step 6 and after you complete step 7, which will create the windows-1250 encoded file, you can rename the out.txt file and go back to step 4.
- Create the decode.go file with the following content:
package main
import (
"fmt"
"io/ioutil"
"os"
"strings"
"golang.org/x/text/encoding/charmap"
)
func main() {
// Open windows-1250 file.
f, err := os.Open("win1250.txt")
if err != nil {
panic(err)
}
defer f.Close()
// Read all in raw form.
b, err := ioutil.ReadAll(f)
if err != nil {
panic(err)
}
content := string(b)
fmt.Println("Without decode: " + content)
// Decode to unicode
decoder := charmap.Windows1250.NewDecoder()
reader := decoder.Reader(strings.NewReader(content))
b, err = ioutil.ReadAll(reader)
if err != nil {
panic(err)
}
fmt.Println("Decoded: " + string(b))
}
- Run the code by executing go run decode.go.
- See the output in the Terminal:

- Create a file with the name encode.go with the following content:
package main
import (
"io"
"os"
"golang.org/x/text/encoding/charmap"
)
func main() {
f, err := os.OpenFile("out.txt", os.O_CREATE|os.O_RDWR,
os.ModePerm|os.ModeAppend)
if err != nil {
panic(err)
}
defer f.Close()
// Decode to unicode
encoder := charmap.Windows1250.NewEncoder()
writer := encoder.Writer(f)
io.WriteString(writer, "Gdańsk")
}
- Run the code by executing go run encode.go.
- See the output in the file out.txt in Windows-1250 encoding and UTF-8 encoding.