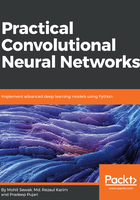
上QQ阅读APP看书,第一时间看更新
Convolutional layers in Keras
To create a convolutional layer in Keras, you must first import the required modules as follows:
from keras.layers import Conv2D
Then, you can create a convolutional layer by using the following format:
Conv2D(filters, kernel_size, strides, padding, activation='relu', input_shape)
You must pass the following arguments:
- filters: The number of filters.
- kernel_size: A number specifying both the height and width of the (square) convolution window. There are also some additional optional arguments that you might like to tune.
- strides: The stride of the convolution. If you don't specify anything, this is set to one.
- padding: This is either valid or same. If you don't specify anything, the padding is set to valid.
- activation: This is typically relu. If you don't specify anything, no activation is applied. You are strongly encouraged to add a ReLU activation function to every convolutional layer in your networks.
It is possible to represent both kernel_size and strides as either a number or a tuple.
When using your convolutional layer as the first layer (appearing after the input layer) in a model, you must provide an additional input_shape argument—input_shape. It is a tuple specifying the height, width, and depth (in that order) of the input.
Please make sure that the input_shape argument is not included if the convolutional layer is not the first layer in your network.
There are many other tunable arguments that you can set to change the behavior of your convolutional layers:
- Example 1: In order to build a CNN with an input layer that accepts images of 200 x 200 pixels in grayscale. In such cases, the next layer would be a convolutional layer of 16 filters with width and height as 2. As we go ahead with the convolution we can set the filter to jump 2 pixels together. Therefore, we can build a convolutional, layer with a filter that doesn't pad the images with zeroes with the following code:
Conv2D(filters=16, kernel_size=2, strides=2, activation='relu', input_shape=(200, 200, 1))
- Example 2: After we build our CNN model, we can have the next layer in it to be a convolutional layer. This layer will have 32 filters with width and height as 3, which would take the layer that was constructed in the previous example as its input. Here, as we proceed with the convolution, we will set the filter to jump one pixel at a time, such that the convolutional layer will be able to see all the regions of the previous layer too. Such a convolutional layer can be constructed with the help of the following code:
Conv2D(filters=32, kernel_size=3, padding='same', activation='relu')
- Example 3: You can also construct convolutional layers in Keras of size 2 x 2, with 64 filters and a ReLU activation function. Here, the convolution utilizes a stride of 1 with padding set to valid and all other arguments set to their default values. Such a convolutional layer can be built using the following code:
Conv2D(64, (2,2), activation='relu')