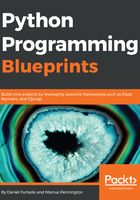
Setting up the environment
Let's go ahead and configure our development environment. The first thing we need to do is create a new virtual environment, so we can work and install the packages that we need without interfering with the global Python installation.
Our application will be called musicterminal, so we can create a virtual environment with the same name.
To create a new virtual environment, run the following command:
$ python3 -m venv musicterminal
And to activate the virtual environment, you can run the following command:
$ . musicterminal/bin/activate
Perfect! Now that we have our virtual environment set up, we can create the project's directory structure. It should have the following structure:
musicterminal
├── client
├── pytify
│ ├── auth
│ └── core
└── templates
Like the application in the first chapter, we create a project directory (called musicterminal here) and a sub-directory also named pytify, which will contain the framework wrapping Spotify's REST API.
Inside the framework directory, we split auth into two modules which will contain implementations for two authentication flows supported by Spotify—authorization code and client credentials. Finally, the core module will contain all the methods to fetch data from the REST API.
The client directory will contain all the scripts related to the client application that we are going to build.
Finally, the templates directory will contain some HTML files that will be used when we build a small Flask application to perform Spotify authentication.
Now, let's create a requirements.txt file inside the musicterminal directory with the following content:
requests==2.18.4
PyYAML==3.12
To install the dependencies, just run the following command:
$ pip install -r requirements.txt

As you can see in the output, other packages have been installed in our virtual environment. The reason for this is that the packages that our project requires also require other packages, so they will also be installed.
Requests were created by Kenneth Reitz https://www.kennethreitz.org/, and it is one of the most used and beloved packages in the Python ecosystem. It is used by large companies such as Microsoft, Google, Mozilla, Spotify, Twitter, and Sony, just to name a few, and it is Pythonic and really straight-forward to use.
Another module that we are going to use is curses. The curses module is simply a wrapper over the curses C functions and it is relatively simpler to use than programming in C. If you worked with the curses C library before, the curses module in Python should be familiar and easy to learn.
One thing to note is that Python includes the curses module on Linux and Mac; however, it is not included by default on Windows. If you are running Windows, the curses documentation at https://docs.python.org/3/howto/curses.html recommends the UniCurses package developed by Fredrik Lundh.
Just one more thing before we start coding. You can run into problems when trying to import curses; the most common cause is that the libncurses are not installed in your system. Make sure that you have libncurses and libncurses-dev installed on your system before installing Python.
If you are using Linux, you will most likely find libncurses on the package repository of our preferred distribution. In Debian/Ubuntu, you can install it with the following command:
$ sudo apt-get install libncurses5 libncurses5-dev
Great! Now, we are all set to start implementing our application.