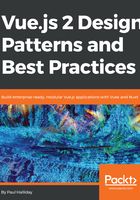
React
React is a JavaScript library developed and maintained by Facebook, and is largely the closest comparison to Vue as their goals are very similar. Like Vue, React is component based and takes advantage of Virtual DOM concepts. This allows for performant rendering of DOM nodes, as a different algorithm is used to determine which parts of the DOM have changed and how best to render/update them on change.
When it comes to templates, React uses JSX to render items on the screen. It takes the more verbose way of creating DOM elements with React.createElement and simplifies it like so:
This is how it will look without JSX:
React.createElement</span>( MyButton, {color: 'red', shadowSize: 5}, 'Click Me' )
Here is how it will look with JSX. As you can see, the two appear very different from one another:
<MyButton color="red" shadowSize={5}>
Click Me
</MyButton>
For newer developers, this adds a slight learning overhead when compared to Vue's simple HTML template, but it is also what gives React its declarative power. It has a state management system using setState(), but also has compatibility with third-party state containers such as Redux and MobX. Vue also has similar capabilities with the Vuex library, and we'll be looking at this in further detail in later sections of this book.
One of the common recent concerns of using React is the BSD + Patents license agreement, something to keep in mind if you're part of an enterprise. Due to this license, Apache recently declared that no Apache software products will use React. Another example of this is the announcement by Wordpress.com that they're no longer using React for their Gutenberg project (https://ma.tt/2017/09/on-react-and-wordpress/). This doesn't necessarily mean that you shouldn't use React in your projects, but is worth pointing out nonetheless.
Some concerned developers elect to use alternatives such as Preact but more recently Vue.js, as it meets a lot of the goals that React developers are looking for when developing applications. To that end, Microsoft (http://dev.office.com/fabric#/components), Apple (https://developer.apple.com/documentation), and countless other companies have products released with React – make of that what you will.