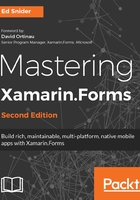
Creating the main page
The main page of the app will serve as the entry point into the app and will display a list of existing trip log entries. Our trip log entries will be represented by a data model named TripLogEntry. Models are a key pillar in the Model-View-ViewModel (MVVM) pattern and data binding, which we will explore more in Chapter 2, MVVM and Data Binding; however, in this chapter, we will create a simple class that will represent the TripLogEntry model.
Let us now start creating the main page by performing the following steps:
- First, add a new Xamarin.Forms XAML ContentPage to the core project and name it MainPage.
- Next, update the MainPage property of the App class in App.xaml.cs to a new instance of Xamarin.Forms.NavigationPage whose root is a new instance of TripLog.MainPage that we just created:
public App()
{
InitializeComponent();
MainPage = new NavigationPage(new MainPage());
}
- Delete TripLogPage.xaml from the core project as it is no longer needed.
- Create a new folder in the core project named Models.
- Create a new empty class file in the Models folder named TripLogEntry.
- Update the TripLogEntry class with auto-implemented properties representing the attributes of an entry:
public class TripLogEntry
{
public string Title { get; set; }
public double Latitude { get; set; }
public double Longitude { get; set; }
public DateTime Date { get; set; }
public int Rating { get; set; }
public string Notes { get; set; }
}
- Now that we have a model to represent our trip log entries, we can use it to display some trips on the main page using a ListView control. We will use a DataTemplate to describe how the model data should be displayed in each of the rows in the ListView using the following XAML in the ContentPage.Content tag in MainPage.xaml:
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="TripLog.MainPage"
Title="TripLog">
<ContentPage.Content>
<ListView x:Name="trips">
<ListView.ItemTemplate>
<DataTemplate>
<TextCell Text="{Binding Title}"
Detail="{Binding Notes}" />
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
</ContentPage.Content>
</ContentPage>
- In the main page's code-behind, MainPage.xaml.cs, we will populate the ListView ItemsSource with a hard-coded collection of TripLogEntry objects. In the next chapter, we will move this collection to the page's data context (that is its ViewModel), and in Chapter 6, API Data Access, we will replace this hard-coded data with data from a live Azure backend:
public partial class MainPage : ContentPage
{
public MainPage()
{
InitializeComponent();
var items = new List<TripLogEntry>
{
new TripLogEntry
{
Title = "Washington Monument",
Notes = "Amazing!",
Rating = 3,
Date = new DateTime(2017, 2, 5),
Latitude = 38.8895,
Longitude = -77.0352
},
new TripLogEntry
{
Title = "Statue of Liberty",
Notes = "Inspiring!",
Rating = 4,
Date = new DateTime(2017, 4, 13),
Latitude = 40.6892,
Longitude = -74.0444
},
new TripLogEntry
{
Title = "Golden Gate Bridge",
Notes = "Foggy, but beautiful.",
Rating = 5,
Date = new DateTime(2017, 4, 26),
Latitude = 37.8268,
Longitude = -122.4798
}
};
trips.ItemsSource = items;
}
}
At this point, we have a single page that is displayed as the app's main page. If we debug the app and run it in a simulator, emulator, or on a physical device, we should see the main page showing the list of log entries we hard-coded into the view, as shown in the following screenshot.

In Chapter 2, MVVM and Data Binding, we will refactor this quite a bit as we implement MVVM and leverage the benefits of data binding.