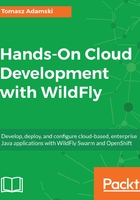
Draft version
We will start by introducing the first, draft version of the service, which we will examine and extend later.
As in the preceding chapter, we have to start with the pom.xml:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.packt.swarm.petstore</groupId>
<artifactId>catalog-service-jaxrs</artifactId>
<version>1.0</version>
<packaging>war</packaging>
(...)
<dependencies>
<!-- 1 -->
<dependency>
<groupId>org.wildfly.swarm</groupId>
<artifactId>jaxrs</artifactId>
<version>${version.wildfly.swarm}</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<artifactId>maven-war-plugin</artifactId>
<version>${version.war.plugin}</version>
<configuration>
<failOnMissingWebXml>false</failOnMissingWebXml>
</configuration>
</plugin>
<!-- 2 -->
<plugin>
<groupId>org.wildfly.swarm</groupId>
<artifactId>wildfly-swarm-plugin</artifactId>
<version>${version.wildfly.swarm}</version>
<executions>
<execution>
<goals>
<goal>package</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
We have to add the dependency to JAX-RS fraction (1) and configure the WildFly Swarm plugin (2). Let's move to the code now.
We will start with a simple domain class, Item, which contains information about the pets available in the store:
package org.packt.swarm.petstore.catalog.model;
public class Item {
private String itemId;
private String name;
private int quantity;
private String description;
public String getItemId() {
return itemId;
}
public void setItemId(String itemId) {
this.itemId = itemId;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
}
As you can see in the preceding code, this is a simple class containing itemId, name, description of the pet, and the quantity available in the store. As in the Hello World example, we have to initialize our JAX-RS application:
package org.packt.swarm.petstore.catalog;
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
@ApplicationPath("/")
public class CatalogApplication extends Application {
}
Finally, we are ready to write a simple JAX-RS resource that will serve up information about available pets from the in-memory HashMap:
package org.packt.swarm.petstore.catalog;
import org.packt.swarm.petstore.catalog.model.Item;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import java.util.HashMap;
import java.util.Map;
//1
@Path("/")
public class CatalogResource {
//2
private Map<String, Item> catalog = new HashMap<>();
public CatalogResource(){
Item turtle = new Item();
turtle.setItemId("turtle");
turtle.setName("turtle");
turtle.setQuantity(5);
turtle.setDescription("Slow, friendly reptile. Let your busy self see how it spends 100 years of his life laying on sand and swimming.");
catalog.put("turtle", turtle);
}
//3
@GET
@Path("item/{itemId}")
@Produces(MediaType.APPLICATION_JSON)
public Response searchById(@PathParam("itemId") String itemId) {
try {
Item item = catalog.get(itemId);
return Response.ok(item).build();
} catch (Exception e) {
return Response.status(Response.Status.BAD_REQUEST).entity(e.getMessage()).build();
}
}
}
Our resource is located at the root path of an application (1). In the first version, we have implemented the catalog as a HashMap and populated it with the first pet—turtle (2). The searchById method will be invoked when the GET method is invoked with the "item" address and the itemId parameter (3).
We can build and deploy the application as in the first chapter:
mvn wildfly-swarm:run
If we enter the address of the catalog-service in the web browser, we will be able to find our first pet in the catalog:
