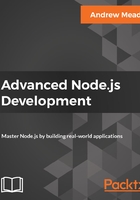
Configuring the Todo and Users file
Inside of models, we're going to create two files, one for each model. I'm going to make two new files called todo.js, and user.js. We can take the todos and Users models from the server.js file and simply copy and paste them into their appropriate files. Once the model's copied, we can remove it from server.js. The Todos model is going to look like this:
var Todo = mongoose.model('Todo', { text: { type: String, required: true, minlength: 1,
trim: true },
completed: {
type: Boolean,
default: false
},
completedAt: {
type: Number,
default: null
}
});
The user.js model is going to look like this.
var User = mongoose.model('User', { email: { type: String, required: true, trim: true, minlength: 1 } });
I'm also going to remove everything we have so far, since those examples in server.js aren't necessary anymore. We can simply leave our mongoose import statement up at the top.
Inside of these model files, there are a few things we need to do. First up, we will call the mongoose.model in both Todos and Users files, so we still need to load in Mongoose. Now, we don't have to load in the mongoose.js file we created; we can load in the plain old library. Let's make a variable. We'll call that variable mongoose, and we're going to require('mongoose'):
var mongoose = require('mongoose');
var Todo = mongoose.model('Todo', {
The last thing that we need to do is export the model, otherwise we can't use it in files that require this one. I'm going to set module.exports equal to an object, and we'll set the Todo property equal to the Todo variable; this is exactly what we did over in mongoose.js:
module.exports = {Todo};
And we're going to do the exact same thing in user.js. Inside of user.js, up at the top, we'll create a variable called mongoose requiring mongoose, and at the bottom we'll export the User model, module.exports, setting it equal to an object where User equals User:
Var mongoose = require('mongoose');
var User = mongoose.model('User', {
email: {
type: String,
required: true,
trim: true,
minlength: 1
}
});
module.exports = {User};
Now, all three of our files have been formatted. We have our three new files and our one old one. The last thing left to do is load in Todo and User.