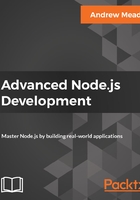
Test case: should not create todo with invalid body data
To get started with this one, we will be using it to create a brand-new test case. The text for this one could be something like should not create todo with invalid body data. We can pass in our callback with the done argument, and start making our super-test request.
This time around, there is no need to make a text variable since we're not going to be passing text into it. What we're going to be doing is passing in nothing at all:
it('should not create todo with invalid body data', (done) => { });
Now, what I'd like you to do is make a request just like we did previously. You're going to make a POST request to the same URL, but instead you're going to send send as an empty object. This empty object is going to cause the test to fail because we won't be able to save the model. Then, you're going to expect we get a 400, which would be the case, we send a 400 in the server.js file. You don't need to make any assumptions about the body that comes back.
Last but not least, you are going to use the following format; we pass a callback to end, check for any errors, and then make some assumptions about the database. The assumption you're going to make is that the length of todos is 0. Since the preceding code block does not create a Todo, no Todos should be there. The beforeEach function is going to run before every test case, so the Todo that gets created in should create a new todo is going to get deleted before our case runs. Go ahead and set that up. Make the request and verify that the length is 0. You don't need to have the assertion in the previous test case, since this assertion asserts something about the array, and the array is going to be empty. You can also leave the following assertion off:
.expect((res) => { expect(res.body.text).toBe(text); })
Since we're not going to make any assertions about the body. When you're done, save the test file. Make sure both of your tests pass.
The first thing I'm going to do is call request, passing in our app. We want to make another post request, so I'll call .post again, and the URL is also going to be the same. Now at this point, we are going to be calling .send, but we're not going to be passing invalid data. The whole point of this test case is to see what happens when we pass in invalid data. What should happen is we should get a 400, so I'm going to expect that a 400 response is what comes back from the server. Now we don't need to make any assertions about the body, so we can go ahead and move on to .end, where we're going to pass in our function that gets called with the err argument, if any, and res, just like this:
it('should not create todo with invalid body data', (done) => { request(app) .post('/todos') .send({}) .expect(400) .end((err, res) => { }); });
Now, we want to do is handle any potential errors. If there is an error we're going to return, which stops the function from executing, and we're going to call done, passing in that error so the test properly fails:
.end((err, res) => { if(err) { return done(err); } });