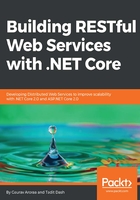
上QQ阅读APP看书,第一时间看更新
Generating the controller
The next step is to add the controller. To do so, refer to the following steps:
- Right-click on the Controller folder, then click on Add, followed by Controller. You will end up on a modal where you will see options to create different types of controllers:

- Select API Controller with actions, using Entity Framework and click on the Add button. The following screenshot shows what happens next:

- Click on Add. Voila! It did all that hard work and created a fully fledged controller using EF Core with actions using all major HTTP verbs. The following code block is a small snapshot of the controller with the GET methods only. I have removed other methods to save space:
// Removed usings for brevity.
namespace DemoECommerceApp.Controllers
{
[Produces("application/json")]
[Route("api/Customers")]
public class CustomersController : Controller
{
private readonly FlixOneStoreContext _context;
public CustomersController(FlixOneStoreContext context)
{
_context = context;
}
// GET: api/Customers
[HttpGet]
public IEnumerable<Customers> GetCustomers()
{
return _context.Customers;
}
// GET: api/Customers/5
[HttpGet("{id}")]
public async Task<IActionResult> GetCustomers
([FromRoute] Guid id)
{
if (!ModelState.IsValid)
{
return BadRequest(ModelState);
}
var customers = await
_context.Customers.SingleOrDefaultAsync(m => m.Id == id);
if (customers == null)
{
return NotFound();
}
return Ok(customers);
}
// You will also find PUT POST, DELETE methods.
// These action methods are removed to save space.
}
}
Some points to note here:
- Notice how FlixOneStoreContext is initialized here by injecting it into the constructor. Further more, it will be used for database-related operations inside all actions:
private readonly FlixOneStoreContext _context;
public CustomersController(FlixOneStoreContext context)
{
_context = context;
}
- The next thing to focus on is the methods used to return the results from the actions. See how BadRequest(), NotFound(), Ok(), and NoContent() are used to return proper HTTP response codes that can be easily understood by clients. We will see what codes they return in a while when we call these actions to perform real tasks.