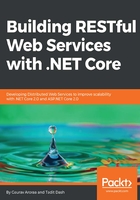
Setting up EF with the API
To use EF Core, the following package is required, which can be downloaded and installed from NuGet Package Manager inside Tools:

Additionally, we need another package named Microsoft.EntityFrameworkCore.Tools. This will help us with creating model classes from the database:

Now, we arrive at the point where we need model classes according to the database tables. The following powershell command can be executed inside the package manager console to create the model class for the Customers table:
Scaffold-DbContext "Server=.;Database=FlixOneStore;Trusted_Connection=True;" Microsoft.EntityFrameworkCore.SqlServer -OutputDir Models -Tables Customers
We have provided the connection string in the command so that it connects to our database.
The following are two important parts of the command we just explored:
- -OutputDir Models: This defines the folder in which the model class will be placed.
- -Tables Customers: This defines the table that will be extracted as the model class. We will be dealing with Customers for now.
After execution, you will see two files, Customers.cs and FixOneStoreContext.cs, inside the Models folder. The Customers.cs file will be something like the following:
using System;
namespace DemoECommerceApp.Models
{
public partial class Customers
{
public Guid Id { get; set; }
public string Gender { get; set; }
public string Firstname { get; set; }
public string Lastname { get; set; }
public DateTime Dob { get; set; }
public string Email { get; set; }
public Guid? Mainaddressid { get; set; }
public string Telephone { get; set; }
public string Fax { get; set; }
public string Password { get; set; }
public bool Newsletteropted { get; set; }
}
}