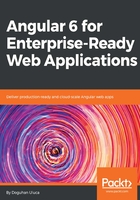
Inspecting and updating package.json
Package.json is the single most important configuration file that you should be keenly aware of at all times. Your project's scripts, runtime, and development dependencies are stored in this file.
- Open package.json and locate the name and version properties:
package.json {
"name": "local-weather-app",
"version": "0.0.0",
"license": "MIT",
...
- Rename your app to whatever you wish; I will be using localcast-weather
- Set your version number to 1.0.0
npm uses semantic versioning (semver), where version number digits represent Major.Minor.Patch increments. Semver starts version numbers at 1.0.0 for any published API, though it doesn't prevent 0.x.x versioning. As the author of a web application, the versioning of your app has no real impact on you, outside of internal tooling, team, or company communication purposes. However, the versioning of your dependencies is highly critical to the reliability of your application. In summary, Patch versions should just be bug fixes. Minor versions add functionality without breaking the existing features, and major version increments are free to make incompatible API changes. However, in reality, any update is risky to the tested behavior of your application. This is why the package-lock.json file stores the entire dependency tree of your application, so the exact state of your application can be replicated by other developers or Continuous Integration servers. For more information, visit: https://semver.org/.
In the following code block, observe that the scripts property contains a collection of helpful starter scripts that you can expand on. The start and test commands are npm defaults, so they can just be executed by npm start or npm test. However, the other commands are custom commands that must be prepended with the run keyword. For example, in order to build your application, you must use npm run build:
package.json ...
"scripts": {
"ng": "ng",
"start": "ng serve",
"build": "ng build",
"test": "ng test",
"lint": "ng lint",
"e2e": "ng e2e"
},
...
Before the introduction of npx, if you wanted to use Angular CLI without a global install, you would have to run it with npm run ng -- g c my-new-component. The double-dashes are needed to let npm know where the command-line tool name ends and options begin. For example, in order to start your Angular application on a port other than the default 4200, you will need to run npm start -- --port 5000.
- Update your package.json file to run your development version of the app from a little used port like 5000 as the new default behavior:
package.json
...
"start": "ng serve --port 5000",
...
Under the dependencies property, you can observe your runtime dependencies. These are libraries that will get packaged up alongside your code and shipped to the client browser. It's important to keep this list to a minimum:
package.json
...
"dependencies": {
"@angular/animations": "^6.0.0",
"@angular/common": "^6.0.0",
"@angular/compiler": "^6.0.0",
"@angular/core": "^6.0.0",
"@angular/forms": "^6.0.0",
"@angular/http": "^6.0.0",
"@angular/platform-browser": "^6.0.0",
"@angular/platform-browser-dynamic": "^6.0.0",
"@angular/router": "^6.0.0",
"core-js": "^2.5.4",
"rxjs": "^6.0.0",
"zone.js": "^0.8.26"
},
...
In the preceding example, all Angular components are on the same version. As you install additional Angular components or upgrade inpidual ones, it is advisable to keep all Angular packages on the same version. This is especially easy to do since npm 5 doesn't require the --save option anymore to permanently update the package version. For example, just executing npm install @angular/router is sufficient to update the version in package.json. This is a positive change overall, since what you see in package.json will match what is actually installed. However, you must be careful, because npm 5 will also automatically update package-lock.json, which will propagate your, potentially unintended, changes to your team members.
Your development dependencies are stored under the devDependencies property. When installing new tools to your project, you must take care to append the command with --save-dev so that your dependency will be correctly categorized. Dev dependencies are only used during development and are not shipped to the client browser. You should familiarize yourself with every single one of these packages and their specific purpose. If you are unfamiliar with a package shown as we move on, your best resource to learn more about them is https://www.npmjs.com/:
package.json
...
"devDependencies": {
"@angular/compiler-cli": "^6.0.0",
"@angular-devkit/build-angular": "~0.6.1",
"typescript": "~2.7.2",
"@angular/cli": "~6.0.1",
"@angular/language-service": "^6.0.0",
"@types/jasmine": "~2.8.6",
"@types/jasminewd2": "~2.0.3",
"@types/node": "~8.9.4",
"codelyzer": "~4.2.1",
"jasmine-core": "~2.99.1",
"jasmine-spec-reporter": "~4.2.1",
"karma": "~1.7.1",
"karma-chrome-launcher": "~2.2.0",
"karma-coverage-istanbul-reporter": "~1.4.2",
"karma-jasmine": "~1.1.1",
"karma-jasmine-html-reporter": "^0.2.2",
"protractor": "~5.3.0",
"ts-node": "~5.0.1",
"tslint": "~5.9.1"
}
...
The characters in front of the version numbers have specific meanings in semver.
- Tilde ~ enables tilde ranges when all three digits of the version number are defined, allowing for patch version upgrades to be automatically applied
- Up-caret character ^ enables caret ranges, allowing for minor version upgrades to be automatically applied
- Lack of any character signals npm to install that exact version of the library on your machine
You may notice that major version upgrades aren't allowed to happen automatically. In general, updating packages can be risky. In order to ensure, no package is updating without your explicit knowledge, you may install exact versions packages by using npm's --save-exact option. Let's experiment with this behavior by installing an npm package that I published called, dev-norms, a CLI tool that generates a markdown file with sensible default norms for your team to have a conversation around, as shown here:
- Under the local-weather-app directory, execute npm install dev-norms --save-dev --save-exact. Note that "dev-norms": "1.3.6" or similar has been added to package.json with package-lock.json automatically updated to reflect the changes accordingly.
- After the tool is installed, execute npx dev-norms create. A file named dev-norms.md has been created containing the aforementioned developer norms.
- Save your changes to package.json.
Working with stale packages comes with its own risks. With npm 6, the npm audit command has been introduced to make you aware of any vulnerabilities discovered in packages you're using. During npm install if you receive any vulnerability notices, you may execute npm audit to find out details about any potential risk.
In the next section, you will commit the changes you have made to Git.