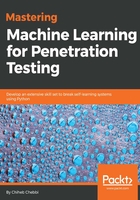
Keras
Keras is a widely used Python library for building deep learning models. It is so easy, because it is built on top of TensorFlow. The best way to build deep learning models is to follow the previously discussed steps:
- Loading data
- Defining the model
- Compiling the model
- Fitting
- Evaluation
- Prediction
Before building the models, please ensure that SciPy and NumPy are preconfigured. To check, open the Python command-line interface and type, for example, the following command, to check the NumPy version:
>>>print numpy.__version__
To install Keras, just use the PIP utility:
$ pip install keras

And of course to check the version, type the following command:
>>> print keras.__version__
To import from Keras, use the following:
from keras import [what_to_use]
from keras.models import Sequential
from keras.layers import Dense
Now, we need to load data:
dataset = numpy.loadtxt("DATASET_HERE", delimiter=",")
I = dataset[:,0:8]
O = dataset[:,8]
#the data is splitted into Inputs (I) and Outputs (O)
You can use any publicly available dataset. Next, we need to create the model:
model = Sequential()
# N = number of neurons
# V = number of variable
model.add(Dense(N, input_dim=V, activation='relu'))
# S = number of neurons in the 2nd layer
model.add(Dense(S, activation='relu'))
model.add(Dense(1, activation='sigmoid')) # 1 output
Now, we need to compile the model:
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
And we need to fit the model:
model.fit(I, O, epochs=E, batch_size=B)
As discussed previously, evaluation is a key step in machine learning; so, to evaluate our model, we use:
scores = model.evaluate(I, O)
print("\n%s: %.2f%%" % (model.metrics_names[1], scores[1]*100))
To make a prediction, add the following line:
predictions = model.predict(Some_Input_Here)