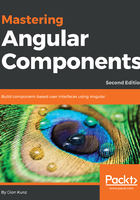
Bootstrapping
The starting point of our project is located within the src/main.ts file. This file is responsible for bootstrapping the Angular framework and starting our applications main module.
We can go ahead and bootstrap our Angular application by providing our main application module, AppModule.
In order to bootstrap an Angular module, we first need to create a platform. There are many ways for different platforms and environments to create a platform. If you'd like to create a browser platform, which is the default platform for browser environments, we need to import the platform factory function platformBrowserDynamic from the @angular/platform-browser-dynamic module. Simply by calling the platform factory function, we will receive an instance of the newly created platform. On the platform instance, we can then call the bootstrapModule function, passing our main application module as a parameter:
import {platformBrowserDynamic} from '@angular/platform-browser-dynamic';
import {AppModule} from './app/app.module';
platformBrowserDynamic().bootstrapModule(AppModule)
.catch(err => console.log(err));
Let's take a closer look at the steps involved in the bootstrapping mechanism of Angular. We should try to understand how our root component is getting rendered in the right place by calling the bootstrapModule function on the platform instance:
- First, we call the bootstrapModule function on our platform instance, passing our main application module as a parameter
- Angular will examine our main application module metadata and find the AppComponent listed in the bootstrap property of the NgModule configuration
- By evaluating the metadata on the AppComponent, looking at the selector property, Angular will know where to render our root component
- The AppComponent is rendered as our root component into the host element found within the index.html file matching the CSS selector in the selector property on the component metadata