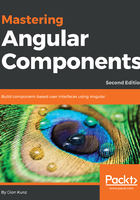
Template strings
Template strings are very simple, but they are an extremely useful addition to the JavaScript syntax. They serve three main purposes:
- Writing multiline strings
- String interpolation
- Tagged template strings
Before template strings, it was quite verbose to write multiline strings. You needed to concatenate pieces of strings and append a new-line character yourself to the line endings:
const header = '<header>\n' +
' <h1>' + title + '</h1>\n' +
'</header>';
Using template strings, we can simplify this example a lot. We can write multiline strings, and we can also use the string interpolation functionality for our title variable that we used to concatenate earlier:
const header = `
<header>
<h1>${title}</h1>
</header>
`;
Note the back ticks instead of the previous single quotes. Template strings are always written between back ticks, and the parser will interpret all characters in-between them as part of the resulting string. This way, the new-line characters present in our source file will also be part of the string automatically.
You can also see that we have used the dollar sign, followed by curly brackets to interpolate our strings. This allows us to write arbitrary JavaScript within strings and helps a lot while constructing HTML template strings.
You can read more about template strings on the Mozilla Developer Network.