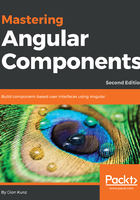
Classes
Classes were among one the most requested features in JavaScript, and I was one of the people voting for it. Well, coming from an OOP background and being used to organizing everything within classes, it was hard for me to let go. Although, after working with modern JavaScript for some time, you'll reduce their use to the bare minimum and to exactly what they are made for—inheritance.
Classes in ECMAScript 6 provide you with syntactic sugar to deal with prototypes, constructor functions, super calls, and object property definitions in a way that you have the illusion that JavaScript could be a class-based OOP language:
class Fruit {
constructor(name) { this.name = name; }
}
const apple = new Fruit('Apple');
As we learned in the previous topic about transpilers, ECMAScript 6 can be de-sugared to ECMAScript 5. Let's take a look at what a transpiler will produce from this simple example:
function Fruit(name) { this.name = name; }
var apple = new Fruit('Apple');
This simple example can easily be built using ECMAScript 5. However, once we use the more complex features of class-based object-oriented languages, the de-sugaring gets quite complicated.
ECMAScript 6 classes introduce simplified syntax to write class member functions (static functions), the use of the super keyword, and inheritance using the extends keyword.
If you would like to read more about the features in classes and ECMAScript 6, I highly recommend that you read the articles of Dr. Axel Rauschmayer (http://www.2ality.com/).