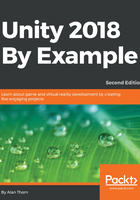
C# scripting in Unity
Defining game logic, rules, and behavior often requires scripting. Specifically, to transform a static and lifeless scene with objects into an environment that does something, a developer needs to code behaviors. It requires someone to define how things should act and react under specific conditions. The coin collection game is no exception to this. In particular, it requires three main features:
- To know when the player collects a coin
- To keep track of how many coins are collected during gameplay
- To determine whether a timer has expired
There's no default out-of-the-box functionality included with Unity to handle this scenario. So we must write some code to achieve it. Unity supports two languages, namely, UnityScript (sometimes called JavaScript) and C#. Both are capable and useful languages, but this book uses C# because, going forward, support for JavaScript will eventually be dropped. Let's start coding these three features in sequence. To create a new script file, right-click on an empty area in the Project panel, and from the context menu, choose Create | C# Script. Alternatively, you can navigate to Assets | Create | C# Script from the application menu. See Figure 2.11:

Figure 2.11: Creating a new C# script
After the file is created, you'll need to assign a descriptive name to it. I'll call it Coin.cs. In Unity, each script file represents a single, discrete class of matching names. Hence, the Coin.cs file encodes the Coin class. The Coin class will encapsulate the behavior of a Coin object and will, eventually, be attached to the Coin object in the scene. See Figure 2.12:

Figure 2.12: Naming a script file
Double-click on the Coin.cs file from the Object Inspector to open it to edit in Visual Studio, a third-party IDE application that ships with Unity. This program lets you edit and write code for your games. Once opened in Visual Studio, the source file will appear, as shown in Code Sample 2.1:
using UnityEngine; using System.Collections; public class Coin : MonoBehaviour { // Use this for initialization void Start () {} // Update is called once per frame void Update () {} }
Note
Downloading the example code
You can download the example code files for this book from GitHub: https://github.com/PacktPublishing/Unity-2018-By-Example-Second-Edition
By default, all newly created classes derive from MonoBehavior, which defines a common set of functionality shared by all components. The Coin class features two autogenerated functions, namely Start and Update. These functions are events invoked automatically by Unity. Start is called once as soon as the GameObject (to which the script is attached) is created in the Scene. Update is called once per frame on the object to which the script is attached. Start is useful for initialization code and Update is useful to create behaviors over time, such as motion and change. Now, before moving any further, let's attach the newly created script file to the Coin object in the Scene. To do this, drag and drop the Coin.cs
script file from the Project panel on the Coin object. When you do this, a new Coin component is added to the object. This means that the script is instantiated and lives on the object. See Figure 2.13, attaching a script file to an object:

Figure 2.13: Attaching a script file to an object
When a script is attached to an object, it exists on the object as a component. A script file can normally be added to multiple objects and even to the same object multiple times. Each component represents a separate and unique instantiation of the class. When a script is attached in this way, Unity automatically invokes its events, such as Start and Update. You can confirm that your script is working normally by including a Debug.Log statement in the Start function.
This prints a debug message to the Console window when the GameObject is created in the Scene. Consider Code Sample 2.2, which achieves this:
using UnityEngine; using System.Collections; public class Coin : MonoBehaviour { // Use this for initialization void Start () { Debug.Log ("Object Created"); } // Update is called once per frame void Update () { } }
If you press play (Ctrl + P) on the toolbar to run your game with the preceding script attached to an object, you will see the message, Object Created, printed to the Console window—once for each instantiation of the class. See Figure 2.14:

Figure 2.14: Printing messages to the Console window
Good work! We've now created a basic script for the Coin class and attached it to the coin. Next, let's define its functionality to keep track of coins as they are collected.