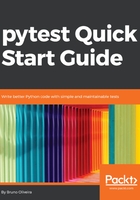
Writing and running tests
Using pytest, all you need to do to start writing tests is to create a new file named test_*.py and write test functions that start with test:
# contents of test_player_mechanics.py
def test_player_hit():
player = create_player()
assert player.health == 100
undead = create_undead()
undead.hit(player)
assert player.health == 80
To execute this test, simply execute pytest, passing the name of the file:
λ pytest test_player_mechanics.py
If you don't pass anything, pytest will look for all of the test files from the current directory recursively and execute them automatically.
Note that there's no need to create classes; just simple functions and plain assert statements are enough, but if you want to use classes to group tests you can do so:
class TestMechanics:
def test_player_hit(self):
...
def test_player_health_flask(self):
...
Grouping tests can be useful when you want to put a number of tests under the same scope: you can execute tests based on the class they are in, apply markers to all of the tests in a class (Chapter 3, Markers and Parametrization), and create fixtures bound to a class (Chapter 4, Fixtures).