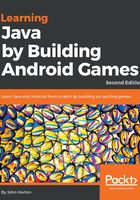
Scope: Methods and Variables
If you declare a variable in a method, whether that is one of the Android methods like onCreate
or one of our own methods it can only be used within that method.
It is no use doing this in onCreate
:
int a = 0;
And, then trying to do this in newGame
or some other method:
a++;
We will get an error because a
is only visible within the method it was declared. At first, this might seem like a problem but perhaps surprisingly, it is actually very useful.
That is why we declared those variables outside of all the methods just after the class declaration. Here they are again as a reminder.
public class SubHunter extends Activity { // These variables can be "seen" // throughout the SubHunter class int numberHorizontalPixels; int numberVerticalPixels; int blockSize; … …
When we do it as we did in the previous code the variables can be used throughout the code file. As this and other projects progress we will declare some variables outside of all methods when they are needed in multiple methods and some variables inside methods when they are needed only in that method.
It is good practice to declare variables inside a method when possible. You can decrease the number of variables coded outside of all methods by using method parameters to pass values around. When you should use each strategy is a matter of design and as the book progresses we will see ways to handle different situations properly.
The term used to describe this topic of whether a variable is usable is the scope. A variable is said to be in scope when it is usable and out of scope when it is not. The topic of scope will also be discussed along with classes in Chapter 8, Object-Oriented Programming.
When we declare variables inside a method we call them local variables. When we declare variables outside of all methods we call them member variables or fields.
Note
A member of what? You might ask. The answer is a member of the class. In the case of the Sub' Hunter project, all those variables are a member of the SubHunter
class as defined by this line of code.
public class SubHunter extends Activity {
Now we can take a closer look at the methods, especially the signatures and return types, that we have already written.