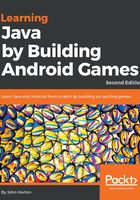
Handling different screen sizes and resolutions
Android is a vast ecosystem of devices and before we can initialize our variables any further we need to know details about the device the game will be running on.
We will write some code to detect the resolution of the screen. The aim of the code when we are done is to store the horizontal and vertical resolutions in our previously declared variables, numberHorizontalPixels,
and numberVerticalPixels
. Also, once we have the resolution information we will also be able to do calculations for initializing gridHeight
and blockSize
.
First, let's get the screen resolution by using some classes and methods of the Android API. Add the highlighted code in the onCreate
method as highlighted next.
/* Android runs this code just before the player sees the app. This makes it a good place to add the code that is needed for the one-time setup. */ @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); // Get the current device's screen resolution Display display = getWindowManager().getDefaultDisplay(); Point size = new Point(); display.getSize(size); Log.d("Debugging", "In onCreate"); newGame(); draw()' }
Tip
What is happening here will become clearer once we have discussed classes further in Chapter 8, Object-Oriented Programming. For now, what follows is a slightly simplistic explanation of the three new lines of code.
The code gets the number of pixels (wide and high) for the device in the following way. Look again at the first new line of code.
Display display = getWindowManager().getDefaultDisplay();
How exactly this works will be explained in more detail in Chapter 11, Collisions, Sound Effects and Supporting Different Android Versions when we discuss chaining. Simply explained, we create an object of type Display
called display
and initialized with the result of calling both getWindowManager
then getDefaultDisplay
methods in turn which are part of the Activity
class.
Then we create a new object called size
of the Point
type. We send size
as an argument to the display.getSize
method. The Point
type has an x
and y
variable already declared, and therefore, so does the size
object, which after the third line of code now holds the width and height (in pixels) of the display.
These values, as we will see next will be used to initialize numberHorizontalPixels
and numberVerticalPixels
.
Also, notice that if you look back to the import
statements at the top of the code the statements relating to the Point
and Display
classes are no longer greyed out because we are now using them.

The explanation just given is necessarily incomplete. Your understanding will improve as we proceed.
Now we have declared all the variables and have stored the screen resolution in the apparently elusive x
and y
variables hidden away in the size
object, we can initialize some more variables and reveal exactly how we get our hands on the variables hidden in size
.