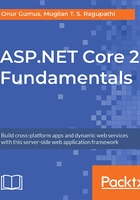
Adding Models
Models represent your business domain classes. Now, we are going to learn about how to use the Models in our controller. Create a Models folder and add a simple Employee class. This is a just a plain old C# class:
Go to https://goo.gl/uBtpw3 to access the code.
public class Employee
{
public int EmployeeId { get; set; }
public string Name { get; set; }
public string Designation { get; set; }
}
Create a new action method, Employee, in our HomeController, and create an object of the Employee Model with some values, and pass the Model to the View. Our idea is to use the Model employee values in the View to present them to the user:
Go to https://goo.gl/r4Jc9x to access the code.
public IActionResult Employee()
{
//Sample Model - Usually this comes from database
Employee emp1 = new Employee
{
EmployeeId = 1,
Name = "Jon Skeet",
Designation = " Software Architect"
};
return View(emp1);
}
Now, we need to add the respective View for this action method. Add a new Razor view file called Employee.cshtml in the View\Home folder.
Add the following code snippet. Whatever comes after the @ symbol is considered as Razor code. In the following code, we are trying to access the properties of the Model object that is passed to our view. In our case, Model represents the employee object that we have constructed in our action method. You can access the object from the view using the Model keyword:
Go to https://goo.gl/u4gCzN to access the code.
<html>
<body>
Employee Name : @Model.Name <br />
Employee Designation: @Model.Designation <br />
</body>
</html>
When you run the application and type the URL http://localhost:50140/Home/ Employee, you'll see the following output:
Alter your View code so that it displays EmployeeID.
Try to display a non-existing property such as @Model.Age. What happens when you do it?
Note that we get an error message if we try to access a non-existing property.