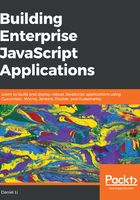
Modularizing our middleware
Let's carry out this refactoring process in a new branch called create-user/refactor-modules:
$ git checkout -b create-user/refactor-modules
Then, create a directory at src/middlewares; this is where we will store all of our middleware modules. Inside it, create four files—one for each middleware function:
$ mkdir -p src/middlewares && cd src/middlewares
$ touch check-empty-payload.js \
check-content-type-is-set.js \
check-content-type-is-json.js \
error-handler.js
Then, move the middleware functions from src/index.js into their corresponding file. For example, the checkEmptyPayload function should be moved to src/middlewares/check-empty-payload.js. Then, at the end of each module, export the function as the default export. For example, the error-handler.js file would look like this:
function errorHandler(err, req, res, next) {
...
}
export default errorHandler;
Now, go back to src/index.js and import these modules to restore the previous behavior:
import checkEmptyPayload from './middlewares/check-empty-payload';
import checkContentTypeIsSet from './middlewares/check-content-type-is-set';
import checkContentTypeIsJson from './middlewares/check-content-type-is-json';
import errorHandler from './middlewares/error-handler';
...
app.use(errorHandler);
Now, run our E2E tests again to make sure that we haven't broken anything. Also, don't forget to commit your code!
$ git add -A && git commit -m "Extract middlewares into modules"
By pulling out the middleware functions, we've improved the readability of our src/index.js file. The intention and flow of our code is apparent because we've named our functions properly—you understand what the functions do from their names. Next, let's do the same with our request handler.