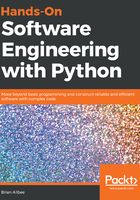
Development paradigms
Programming, when it first appeared, was often limited by hardware capabilities and the higher-level languages that were available at the time for simple procedural code. A program, in that paradigm, was a sequence of steps, executed from beginning to end. Some languages supported subroutines and perhaps even simple function-definition capabilities, and there were ways to, for example, loop through sections of the code so that a program could continue execution until some termination condition was reached, but it was, by and large, a collection of very brute-force, start-to-finish processes.
As the capabilities of the underlying hardware improved over time, more sophisticated capabilities started to become more readily available—formal functions as they are generally thought of now, are more powerful , or at least have a flexible loop and other flow control options, and so on. However, outside a few languages that were generally accessible only inside the halls and walls of Academia, there weren't many significant changes to that procedural approach in mainstream efforts until the 1990s, when Object-Oriented Programming first started to emerge as a significant, or even dominant paradigm.
The following is an example of a fairly simple procedural program that asks for a website URL, reads the data from it, and writes that data to a file:
#!/usr/bin/env python
"""
An example of a simple procedural program. Asks the user for a URL,
retrieves the content of that URL (http:// or https:// required),
writes it to a temp-file, and repeats until the user tells it to
stop.
"""
import os
import urllib.request
if os.name == 'posix':
tmp_dir = '/tmp/'
else:
tmp_dir = 'C:\\Temp\\'
print('Simple procedural code example')
the_url = ''
while the_url.lower() != 'x':
the_url = input(
'Please enter a URL to read, or "X" to cancel: '
)
if the_url and the_url.lower() != 'x':
page = urllib.request.urlopen(the_url)
page_data = page.read()
page.close()
local_file = ('%s%s.data' % (tmp_dir, ''.join(
[c for c in the_url if c not in ':/']
)
)).replace('https', '').replace('http', '')
with open(local_file, 'w') as out_file:
out_file.write(str(page_data))
print('Page-data written to %s' % (local_file))
print('Exiting. Thanks!')