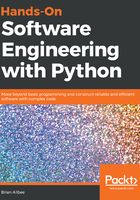
Interprocess communication
It's very common for different processes to communicate with each other. At the most basic level, that communication might take the form of something as simple as one function or method calling another from somewhere in the code they share. As processes scale outward, though, especially if they are distributed across separate physical or virtual devices, those communication chains will often get more complex themselves, sometimes even requiring dedicated communications protocols. Similar communication-process complexities can also surface, even in relatively uncomplicated systems, if there are interprocess dependencies that need to be accounted for.
In pretty much any scenario where the communication mechanism between two processes is more complicated than something at the level of methods calling other methods, or perhaps a method or process writing data that another process will pick up and run with the next time it's executed, it's worth documenting how those communications will work. If the basic unit of communication between processes is thought of as a message, then, at a minimum, documenting the following will generally provide a solid starting point for writing the code that implements those interprocess communication mechanisms:
- What the message contains: The specific data expected:
- What is required in the message
- What additional/optional data might be present
How the message is formatted: If the message is serialized in some fashion, converted to JSON, YAML, or XML, for example, that needs to be noted
How the message is transmitted and received: It could be queued up on a database, transmitted directly over some network protocol, or use a dedicated message-queue system such as RabbitMQ, AWS SQS, or Google Cloud Platform's Publish/Subscribe
What kinds of constraint apply to the message protocol: For example, most message-queuing systems will guarantee the delivery of any given queued message once, but not more than once.
How messages are managed on the receiving end: In some distributed message-queue systems – certain variants of AWS SQS, for example – the message has to be actively deleted from the queue, lest it be received more than once, and potentially acted upon more than once. Others, such as RabbitMQ, automatically delete messages as they are retrieved. In most other cases, the message only lives as long as it takes to reach its destination and be received.
Interprocess-communication diagramming can usually build on the logical architecture and use-case diagrams. One provides the logical components that are the endpoints of the communication process, the other identifies what processes need to communicate with each other. Documented data flow may also contribute to the bigger picture, and would be worth looking at from the perspective of identifying any communication paths that might've been missed elsewhere.
The refuel tracker, for example:
Can access the database for the existing route-scheduling application, which provides a dashboard for the route schedulers.
The maintenance alert functionality can leverage a web service call belonging to an off-the-shelf fleet-maintenance system that was purchased, which has its own dashboard used by the fleet technicians.
The relevant messaging involved for the route-review and maintenance-alert processes is very simple under these circumstances:
An update in the route-scheduling database, perhaps flagging the last route that the truck was scheduled for as an inefficient route, or maybe some sort of notification that'll pop up on the dashboard to alert a route scheduler to review the route
A JSON-over-REST API call made to the maintenance-tracking system
That messaging would fit on a simple variant of the use case diagram already shown:
The order-processing, fulfillment, and shipping system might use RabbitMQ messaging to deal with order-fulfillment, passing entire orders and simple inventory checks from the products datasource to determine whether an order can be fulfilled. It might also use any of several web service API calls to manage order shipment, pushing the shipping information back into the order over a similar web service call. That message flow (omitting the data structure for brevity) might then look as follows: