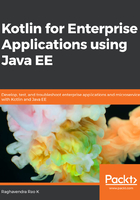
Kotlin in an existing Java project
Let's look at an example of how to add Kotlin code to an existing Java project created using Maven. We have created a simple Maven project that has some code written in Java. This is shown here:
We can add Kotlin code to it as follows.
- Right-click on src/main/java and then click on New | Kotlin File/Class. We call it NewApp.kt:
- After creating a Kotlin file, IDEA prompts us to configure the Kotlin runtime:
- Click on Configure and choose Maven.
- Choose the desired Kotlin version and hit the OK button. We have now configured Kotlin runtime in a Java project.
- The App.java and NewApp.kt files are hello world classes. This means that they just print a hello world message to the console, shown as follows:
App.java:
public class App {
public static void main (String[] args) {
System.out.println ("Hello World from Java!");
}
}
NewApp.kt:
object NewApp {
@JvmStatic
fun main(args: Array<String>) {
println("Hello World from Kotlin!")
}
}
We can also write the main function without the class structure, as follows:
fun main(vararg args: String) {
println("Hello World from Kotlin!")
}
Also, note that we can use vararg for arguments to main function. vararg is followed by the argument name and the type.
Let's run both the Java and Kotlin codes now. The output from the Java code will appear as follows:
The output from the Kotlin code will appear as follows:
As we can see, both Kotlin and Java can exist in the same project, and we configured the Kotlin runtime in a Java project. We can also load and use any Java class or a library written in Java from a Kotlin class.