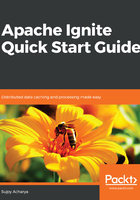
Running HelloWorld
You have successfully installed Apache Ignite, now it's time for fun. Let's connect to the Apache Ignite node and create a cache. The following are the steps to create a new Ignite cache:
- Open your favorite IDE and create a new Gradle project, hello-world
- Edit the build.gradle file with the following entries:
implementation 'com.h2database:h2:1.4.196'
compile group: 'org.apache.ignite', name: 'ignite-core', version:
'2.5.0'
compile group: 'org.slf4j', name: 'slf4j-api', version: '1.6.0'
compile group: 'org.apache.ignite', name: 'ignite-spring',
version: '2.5.0'
compile group: 'org.apache.ignite', name: 'ignite-indexing',
version: '2.5.0'
compile group: 'log4j', name: 'log4j', version: '1.2.17'
- Create a new Java class, HelloWorld
- Add the following lines to create a cache, myFirstIgniteCache
,
put values into the cache, and then retrieve values from the cache:
public class HelloWorld {
public static void main(String[] args) {
try (Ignite ignite = Ignition.start()) {
IgniteCache<Integer, String> cache =
ignite.getOrCreateCache("myFirstIgniteCache");
for (int i = 0; i < 10; i++)
cache.put(i, Integer.toString(i));
for (int i = 0; i < 10; i++)
System.out.println("Fetched [key=" + i + ", val=" +
cache.get(i) + ']');
}
}
}
The Ignition.start() starts an Ignite instance in memory. A cache stores key-value pairs like java.util.Map. IgniteCache<Key, Value> represents a distributed cache where Key and Value are serializable objects. Here, we are asking Ignite to create (or get, if already created) a cache myFirstIgniteCache to store an integer key and String value, then store 10 integers in the cache, and finally ask the cache to get the values:
- Add the import statements and run the program.
- It will start a server node and add it to the existing cluster. You can see the topology snapshot indicating version=2 and servers=2:
Don't panic if the code doesn't look familiar; we will explore it step by step in Chapter 2, Understanding the Topologies and Caching Strategies.