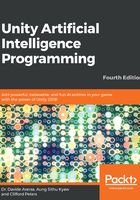
The Bullet class
Next, we set up our Bullet prefab with two orthogonal planes using a laser-like material and a Particles/Additive property in the Shader field:
The code in the Bullet.cs file is as follows:
using UnityEngine; using System.Collections; public class Bullet : MonoBehaviour { //Explosion Effect
[SerializeField] private GameObject Explosion;
[SerializeField]
private float Speed = 600.0f;
[SerializeField]
private float LifeTime = 3.0f;
public int damage = 50; void Start() { Destroy(gameObject, LifeTime); } void Update() { transform.position += transform.forward * Speed * Time.deltaTime; } void OnCollisionEnter(Collision collision) { ContactPoint contact = collision.contacts[0]; Instantiate(Explosion, contact.point, Quaternion.identity); Destroy(gameObject); } }
Our Bullet has three properties: damage, Speed, and Lifetime—the latter so that the bullet will be automatically destroyed after a certain amount of time. Note that we use [SerializeField] to show the private fields in the inspector; by defult, in fact, Unity only shows public fields. It is a good practice to set as public only fields that are used in other classes.
As you can see, the Explosion property of the bullet is linked to the ParticleExplosion prefab, which we're not going to discuss in detail. There's a prefab called ParticleExplosion under the ParticleEffects folder. We just drop that prefab into this field. This particle effect is played when the bullet hits something, as described in the OnCollisionEnter method. The ParticleExplosion prefab uses a script called AutoDestruct to destroy the Explosion object automatically after a small amount of time.
using UnityEngine;
public class AutoDestruct : MonoBehaviour
{
[SerializeField]
private float DestructTime = 2.0f;
void Start()
{
Destroy(gameObject, DestructTime);
}
}
The AutoDestruct is a small but convenient script. It just destroys the attached object after a certain amount of seconds. Unity games use a similar script almost every time for many situations.