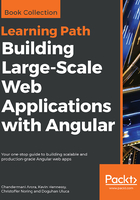
The @Output decorator
We covered a decent amount of Angular eventing capabilities in this chapter. Specifically, we learned how we can consume any event on a component, directive, or DOM element using the bracketed () syntax. How about raising our own events?
In Angular, we can create and raise our own events, events that signify something noteworthy has happened in our component/directive. Using the @Output decorator and the EventEmitter class, we can define and raise custom events.
Remember this: it is through events that components can communicate with the outside world. When we declare:
@Output() exercisePaused: EventEmitter<number> = new EventEmitter<number>();
It signifies that WorkoutRunnerComponent exposes an event, exercisePaused (raised when the workout is paused).
To subscribe to this event, we can do the following:
<abe-workout-runner (exercisePaused)="onExercisePaused($event)"></abe-workout-runner>
This looks absolutely similar to how we did the DOM event subscription in the workout runner template. See this sample stipped from the workout-runner's view:
<div id="pause-overlay" (click)="pauseResumeToggle()" (window:keyup)="onKeyPressed($event)">
The @Output decorator instructs Angular to make this event available for template binding. Events created without the @Output decorator cannot be referenced in HTML.
Like any decorator, the @Output decorator is there just to provide metadata for the Angular framework to work with. The real heavy lifting is done by the EventEmitter class.