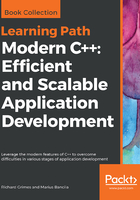
Deleting the task list
Iterating through the list is simple, you follow the pNext pointer from one link to the next. Before doing this, let's first fix the memory leak introduced in the last section. Above the main function, add the following function:
bool remove_head()
{
if (nullptr == pHead) return false;
task* pTask = pHead;
pHead = pHead->pNext;
delete pTask;
return (pHead != nullptr);
}
This function will remove the link at the beginning of the list and make sure that the pHead pointer points to the next link, which will become the new beginning of the list. The function returns a bool value indicating if there are any more links in the list. If this function returns false then it means the entire list has been deleted.
The first line checks to see if this function has been called with an empty list. Once we are reassured that the list has at least one link, we create a temporary copy of this pointer. The reason is that the intention is to delete the first item and make pHead point to the next item, and to do that we have to do those steps in reverse: make pHead point to the next item and then delete the item that pHead previously pointed to.
To delete the entire list, you need to iterate through the links, and this can be carried out using a while loop. Below the remove_head function, add the following:
void destroy_list()
{
while (remove_head());
}
To delete the entire list, and address the memory leak, add the following line to the bottom of the main function
destroy_list();
}
You can now compile the code, and run it. However, you'll see no output because all the code does is create a list and then delete it.