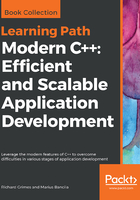
Pointers to Deallocated Memory
This applies to memory allocated on the stack and to memory dynamically allocated. The following is a poorly written function that returns a string allocated on the stack in a function:
char *get()
{
char c[] { "hello" };
return c;
}
The preceding code allocates a buffer of six characters and then initializes it with the five characters of the string literal hello, and the NULL termination character. The problem is that once the function finishes the stack frame is torn down so that the memory can be re-used, and the pointer will point to memory that could be used by something else. This error is caused by poor programming, but it may not be as obvious as in this example. If the function uses several pointers and performs a pointer assignment, you may not immediately notice that you have returned a pointer to a stack-allocated object. The best course of action is simply not to return raw pointers from functions, but if you do want to use this style of programming, make sure that the memory buffer is passed in through a parameter (so the function does not own the buffer) or is dynamically allocated and you are passing ownership to the caller.
This leads on to another issue. If you call delete on a pointer and then later in your code, try to access the pointer, you will be accessing memory that is potentially being used by other variables. To alleviate this problem, you can get into the habit of assigning a pointer to null_ptr when you delete it and check for null_ptr before using a pointer. Alternatively, you can use a smart pointer object which will do this for you. Smart pointers will be covered in Chapter 4, Classes.