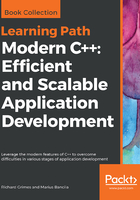
Passing Multidimensional Arrays to Functions
You can pass a multidimensional array to a function:
// pass the torque of the wheel nuts of all wheels
bool safe_torques(double nut_torques[4][5]);
This compiles and you can access the parameter as a 4x5 array, assuming that this vehicle has four wheels with five nuts on each one.
As stated earlier, when you pass an array, the first dimension will be treated as a pointer, so while you can pass a 4x5 array to this function, you can also pass a 2x5 array and the compiler will not complain. However, if you pass a 4x3 array (that is, the second dimension is not the same as declared in the function), the compiler will issue an error that the array is incompatible. The parameter may be more accurately described as being double row[][5]. Since the size of the first dimension is not available, the function should be declared with the size of that dimension:
bool safe_torques(double nut_torques[][5], int num_wheels);
This says that nut_torques is one or more "rows", each of which has five items. Since the array does not provide information about the number of rows it has, you should provide it. Another way to declare this is:
bool safe_torques(double (*nut_torques)[5], int num_wheels);
The brackets are important here, if you omit them and use double *nut_torques[5], then it means the * will refer to the type in the array, that is, the compiler will treat nut_torques as a five-element array of double* pointers. We have seen an example of such an array before:
void main(int argc, char *argv[]);
The argv parameter is an array of char* pointers. You can also declare the argv parameter as char** which has the same meaning.
In general, if you intend to pass arrays to a function it is best to use custom types, or use the C++ array types.
Using ranged for with multidimensional arrays is a bit more complicated than appears on first sight, and requires the use of a reference as explained in the section later in this chapter.